Learn how to efficiently convert CSV to JSON format.
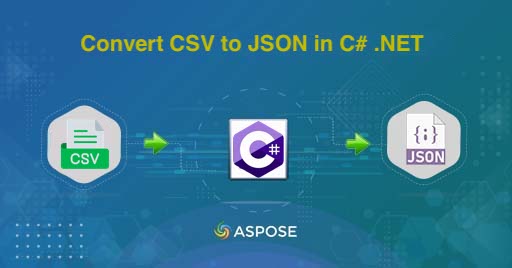
Convert CSV to JSON files in C# .NET
CSV (Comma-Separated Values) is a widely used file format for storing and exchanging tabular data. While CSV is a simple and easy-to-use format, it is not always the most efficient format for web applications. JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate. JSON is increasingly used as a data format for web APIs, making it a popular choice for developers. Converting CSV files to JSON format can improve data processing efficiency and enable data to be consumed by web applications in a more user-friendly manner. In this tutorial, we will guide you through the process of converting CSV files to JSON format using C# .NET.
CSV to JSON Conversion API
Aspose.Cells Cloud SDK for .NET provides several benefits that make it an ideal tool for converting CSV to JSON format. First and foremost, it is a cloud-based API, which means that there is no need to install any software or libraries on your local machine. This makes it easy to get started with, and eliminates the need for complex setup and configuration. Additionally, Aspose.Cells Cloud SDK for .NET is highly scalable and can handle large volumes of data, making it suitable for enterprise-level applications. The conversion process is fast, reliable, and produces high-quality JSON output that is easy to parse and use in web applications.
We will begin by adding the SDK reference in our application via NuGet package manager. Search “Aspose.Cells-Cloud” and click the Add Package button. Secondly, if you do not have an account over Cloud Dashboard, please create a free account by using a valid email address and obtain your personalized credentials.
Convert CSV to JSON using C#
In order to perform the document conversion, we have three API calls to accomplish this requirement.
- GetWorkbook - Get input CSV from Cloud storage. After conversion, save output to cloud storage.
- PutConvertWorkbook - Converts CSV file to other formats from request content.
- PostWorkbookSaveAs - Saves CSV file as other formats file to storage.
In the following code snippet, we are going to use GetWorkbook API, which loads the input CSV from cloud storage, converts it to JSON format and saves the output to the same cloud storage.
Let’s develop our understanding regarding above code snippet:
CellsApi cellsInstance = new CellsApi(clientID, clientSecret);
Create an object of CellsApi while passing client credentials as arguments.
cellsInstance.UploadFile(input_CSV, File.OpenRead(input_CSV));
Upload the input CSV to cloud storage.
var response = cellsInstance.CellsWorkbookGetWorkbook(input_CSV, null, format:"JSON", null, outPath:resultant_File);
Initialize the CSV to JSON conversion operation. After successful conversion, the output JSON file is saved to cloud storage.
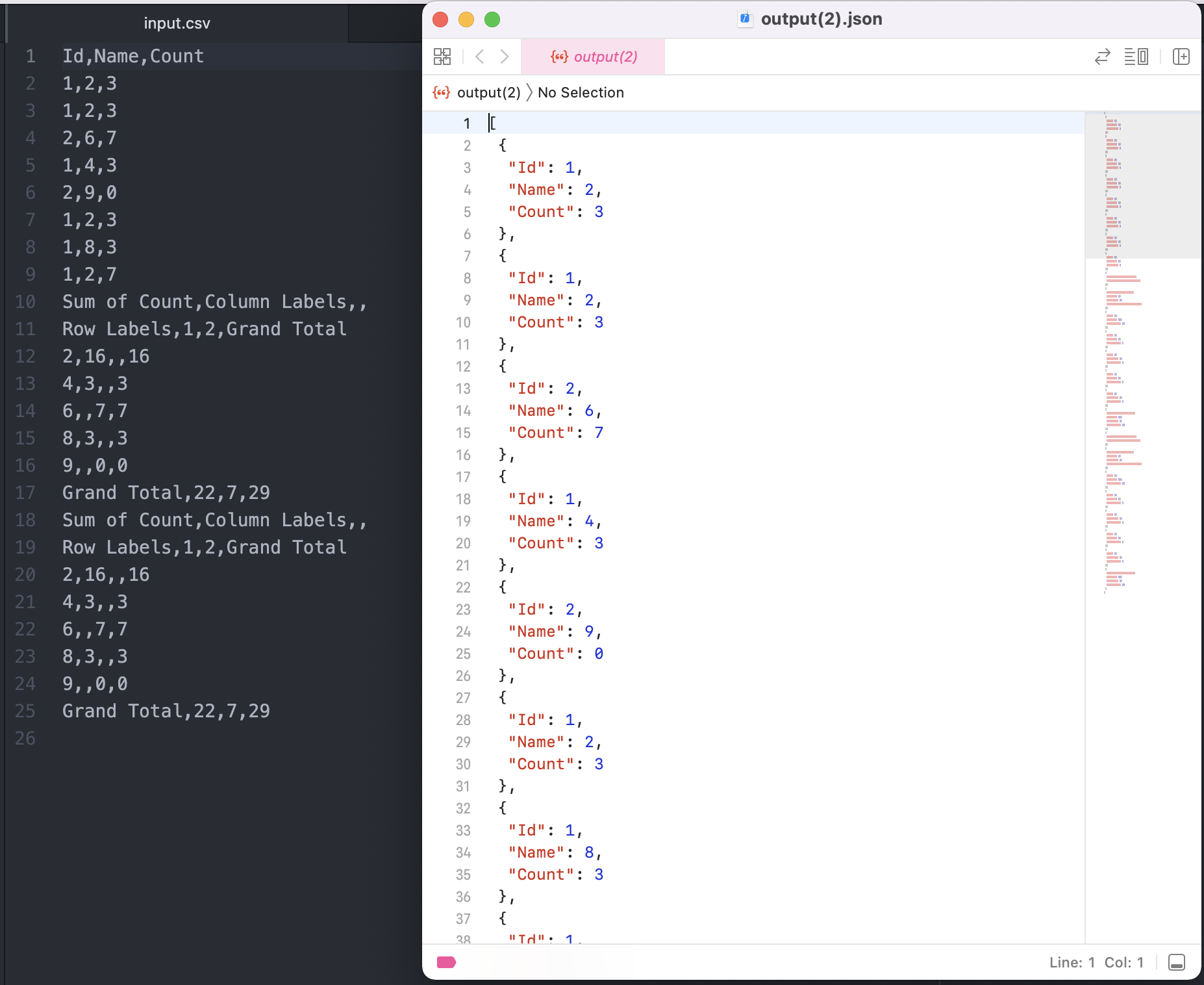
CSV to JSON conversion preview.
The sample CSV used in the above example can be downloaded from input.csv and the resultant JSON file can be downloaded from output.json.
Online CSV to JSON using cURL Commands
Converting CSV to JSON using cURL commands and REST API provides several benefits. First and foremost, it is a simple and easy-to-use approach that requires no additional software or libraries to be installed. Additionally, cURL commands and REST API are platform-independent, which means that the same approach can be used on any operating system or programming language that supports cURL commands and REST API. This makes it an ideal solution for developers who are working with multiple platforms and programming languages.
Now in this section, we are going to learn the steps on how to convert CSV to JSON online using the cURL commands. So the first step is to generate a JWT access token based on the client credentials:
curl -v "https://api.aspose.cloud/connect/token" \
-X POST \
-d "grant_type=client_credentials&client_id=bb959721-5780-4be6-be35-ff5c3a6aa4a2&client_secret=4d84d5f6584160cbd91dba1fe145db14" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "Accept: application/json"
Once we have the JWT token, we are going to call GetWorkbook API to convert CSV to JSON online. Please execute the following command:
curl -v -X GET "https://api.aspose.cloud/v3.0/cells/input.csv?format=JSON&isAutoFit=false&onlySaveTable=false&outPath=resultant.json&checkExcelRestriction=true" \
-H "accept: application/json" \
-H "<JWT Token>"
Concluding Remarks
In this tutorial, we’ve explored two approaches for converting CSV files to JSON format - using C# .NET and cURL commands with REST API. Both approaches have their advantages, and the choice ultimately depends on the specific needs of your project. With C# .NET, we were able to use the Aspose.Cells Cloud SDK to efficiently convert CSV files to JSON format online, while cURL commands and REST API provided a simple and platform-independent approach that requires no additional software or libraries. Regardless of the approach you choose, converting CSV files to JSON format can bring efficiency and user-friendliness to your web applications, allowing you to streamline data processing and management.
Useful Links
Recommended Articles
Please visit the following links to learn more about: