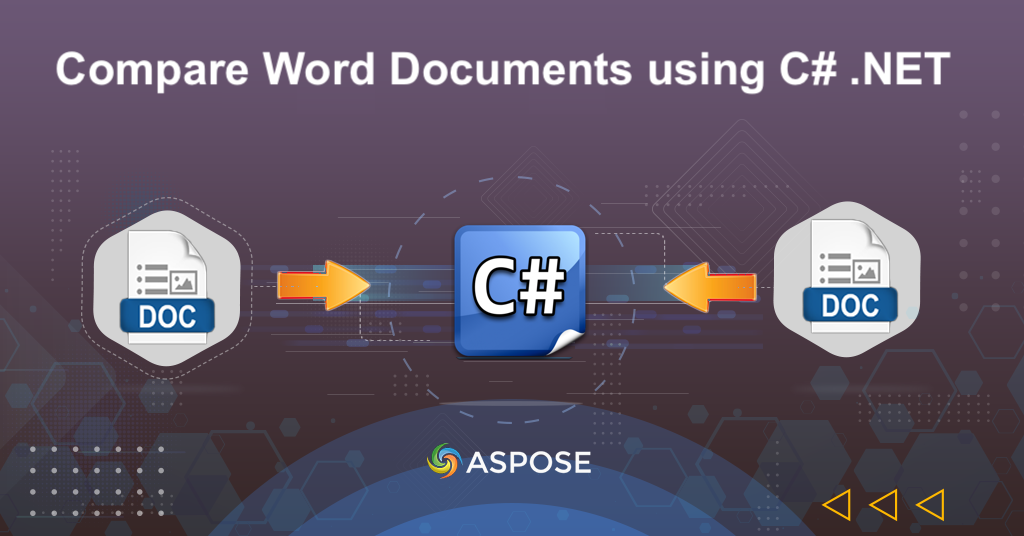
Compare Word Documents using C# .NET
As businesses and individuals rely more on digital documentation, the need for efficient document comparison tools has become increasingly important. Manual comparison of documents can be tedious, time-consuming, and error-prone. Thankfully, with the power of C# .NET, you can automate the process of comparing Word documents, making it faster, more accurate, and more reliable. In this blog post, we’ll explore how you can leverage .NET Cloud SDK to compare Word documents, and how you can make use of its features to save time and improve your document review process.
- REST API to Compare Documents
- Compare Word Documents in C#
- Compare Two Documents using cURL Commands
REST API to Compare Documents
Aspose.Words Cloud SDK for .NET is a powerful tool that can help you compare Word documents with ease. With its comprehensive set of features, you can compare two or more documents, identify changes and differences between them, and generate a report that highlights the changes. Whether you’re a developer, a project manager, or an editor, this SDK can help you streamline your document comparison process and save you valuable time.
Now, in order to use the SDK, please search Aspose.Words-Cloud
in NuGet packages manager and click the Add Package button to add the reference of SDK in .NET project.
Compare Word Documents in C#
Please try using the following code snippet to compare Word documents online.
// create configuration object using ClientID and Client Secret details
var config = new Configuration { ClientId = clientID, ClientSecret = clientSecret };
// initialize WordsApi instance
var wordsApi = new WordsApi(config);
Create an instance of WordsApi using client credentials.
// read the content of first Word document from local drive
var firstFile = System.IO.File.OpenRead(firstDocument);
wordsApi.UploadFile(new UploadFileRequest(firstFile, firstDocument));
Read the content of input Word document and upload to Cloud storage.
CompareData compareData = new CompareData();
compareData.ComparingWithDocument = secondDocument;
compareData.Author = "Nayyer Shahbaz";
compareData.DateTime = DateTime.Now.Date;
compareData.ResultDocumentFormat = "DOCX";
Create an instance of CompareData object where we specify the name of document to be compared, author details, date & time information and the format for the resultant document.
CompareOptions options = new CompareOptions()
{
IgnoreCaseChanges = true,
IgnoreFormatting = true,
IgnoreHeadersAndFooters = true,
IgnoreFootnotes = true,
IgnoreComments = true,
IgnoreTextboxes = true,
IgnoreTables = true,
Target = CompareOptions.TargetEnum.Current,
AcceptAllRevisionsBeforeComparison = true
};
compareData.CompareOptions = options;
Create CompareOptions instance where we define various comparison options.
CompareDocumentRequest compare = new CompareDocumentRequest();
compare.CompareData = compareData;
compare.Name = firstDocument;
compare.DestFileName = resultantFile;
Specify the CompareData instance, name of source Word document and the name of output file containing comparison result.
wordsApi.CompareDocument(compare);
Initiate the document comparison operation and generate the output in Cloud storage.
Compare Two Documents using cURL Commands
We have discussed that comparing Word documents can be a challenging and time-consuming task, especially when dealing with multiple files or complex formatting. Fortunately, Aspose.Words Cloud and cURL commands provide a powerful solution for comparing Word documents quickly and easily. We can compare two or more documents, track changes, and generate a detailed report that highlights differences between the documents.
Now, please execute the following command to create a JWT access token based on Client ID and Client Secret details.
curl -v "https://api.aspose.cloud/connect/token" \
-X POST \
-d "grant_type=client_credentials&client_id=a41d01ef-dfd5-4e02-ad29-bd85fe41e3e4&client_secret=d87269aade6a46cdc295b711e26809af" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "Accept: application/json"
Once the JWT Token has been generated, please execute the following command to compare two Word documents online and, save the resultant file in cloud storage.
curl -v "https://api.aspose.cloud/v4.0/words/{sourceFirst}/compareDocument?destFileName={differenceFile}" \
-X PUT \
-H "accept: application/json" \
-H "Authorization: Bearer {accessToken}" \
-H "Content-Type: application/json" \
-d "{\"ComparingWithDocument\":\"input-sample-2.docx\",\"Author\":\"Nayyer Shahbaz\",\"DateTime\":\"2023-04-23T06:02:29.481Z\",\"CompareOptions\":{\"IgnoreCaseChanges\":true,\"IgnoreTables\":true,\"IgnoreFields\":true,\"IgnoreFootnotes\":true,\"IgnoreComments\":true,\"IgnoreTextboxes\":true,\"IgnoreFormatting\":true,\"IgnoreHeadersAndFooters\":true,\"Target\":\"Current\",\"AcceptAllRevisionsBeforeComparison\":true},\"ResultDocumentFormat\":\"docx\"}"
Replace
{sourceFirst}
with the name of input Word document (present in the Cloud storage),{accessToken}
with JWT access token generated above and{differenceFile}
with the name of resultant Word document highlighting the comparison difference.
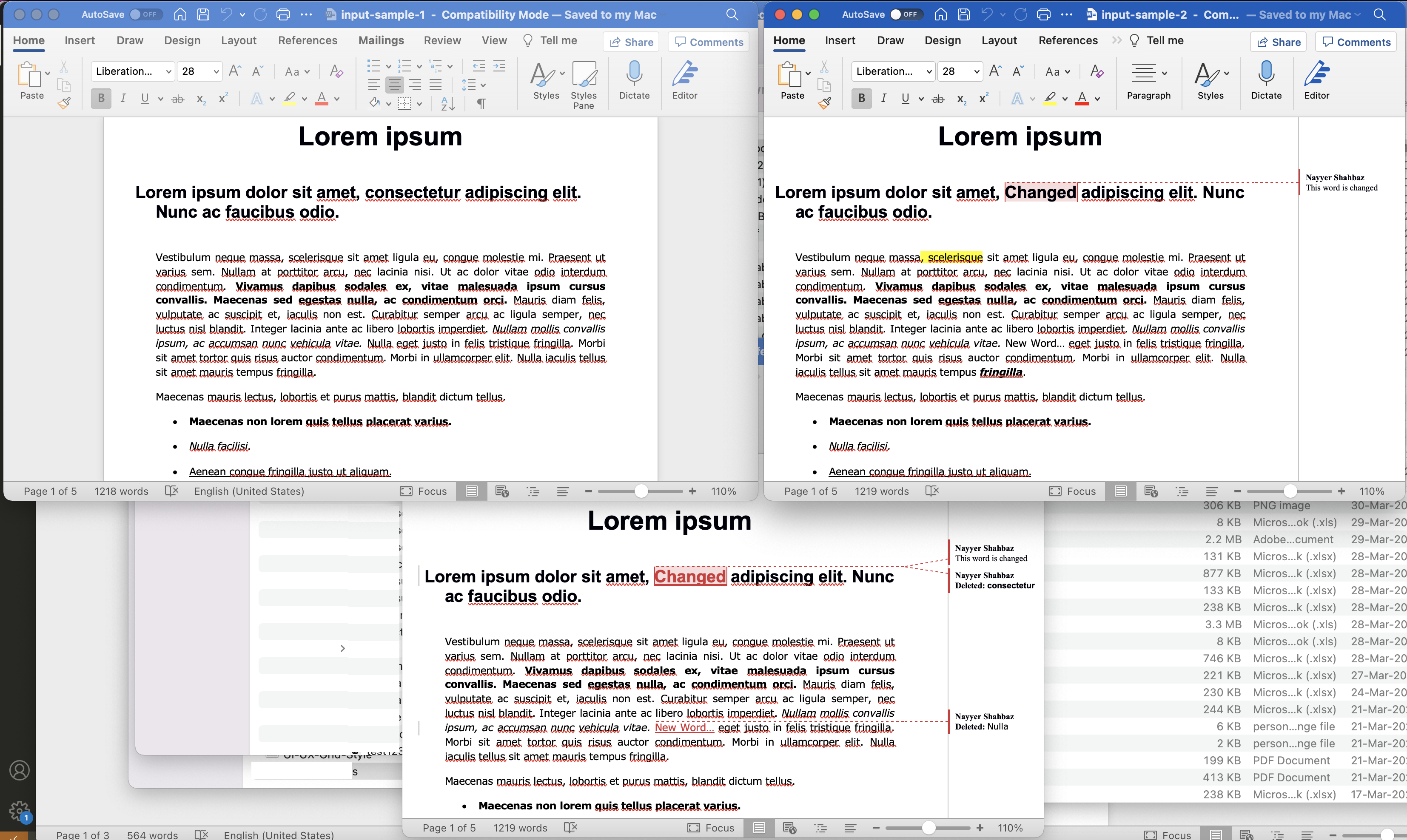
Image:- Preview of Word document comparison using .NET REST API.
Conclusion
In conclusion, comparing Word documents can be a daunting task, but with the help of Aspose.Words Cloud and cURL commands or its robust .NET Cloud SDK, you have a powerful solution at your disposal. Whether you prefer to work with C# .NET or prefer to use command line tools such as cURL, Aspose.Words Cloud offers a range of options for comparing two or more documents, tracking changes, and generating detailed reports. With its flexible and customizable features, it is an essential resource for anyone who works with Word documents on a regular basis. So why not give it a try and see how it can help you streamline your document comparison process.
Useful Links
Related Articles
We highly recommend going through the following blogs: