Develop online JPG to Word converter with few code lines. Your JPG to DOC Converter using Python SDK.
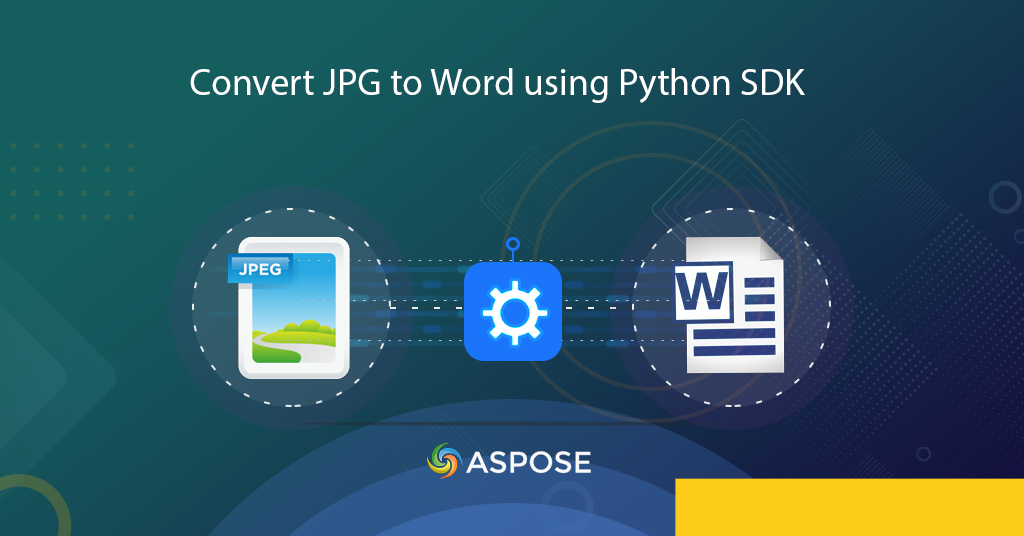
Convert JPG to Word | JPG to DOC converter using Python SDK
This article explains the steps for developing JPG to Word converter using a Cloud-based API. No software download or installation is required and perform all conversion operations using REST API calls. We understand that raster image formats especially JPG are one of the most widely used formats for pictures/images sharing. Also, JPEG is a commonly used method of lossy compression for digital images, particularly for those images produced by digital photography. The degree of compression can be adjusted, allowing a selectable tradeoff between storage size and image quality. However, we may have a requirement to combine multiple related images in a single file so either we use an approach to Merge JPG images online using C# REST API but another solution can be the placement of JPG images inside a word document and save them in a document repository. Now let’s further explore the details of how we can develop a JPG to DOC converter using Python programming language.
Please note that in order to perform the conversion, we need to use two
PDF Conversion API
Aspose.PDF Cloud is a REST architecture-based solution providing the capabilities to create, edit and convert various file formats (EPUB, HTML, XML, XPS, Text, etc) to PDF and similarly, supports the conversion of PDF documents to JPEG, DOC, XLS, PPTX, etc) format. As per the scope of this article, we are going to insert JPG images inside a PDF file and then transform it into MS Word (DOC) format using Python SDK. So the first step is the installation of Aspose.PDF Cloud SDK for Python, which is a wrapper around Cloud API so that you get all the features within your favorite Python IDE.
Requirements
The Cloud SDK requires Python 2.7 and 3.4+
Installation
pip install
If the python package is available on Github, you can install it directly from Github
pip install git+https://github.com/aspose-pdf-cloud/aspose-pdf-cloud-python.git
(you may need to run pip
with root permission:
sudo pip install git+https://github.com/aspose-pdf-cloud/aspose-pdf-cloud-python.git
Setuptools
Install via Setuptools.
python setup.py install --user
After the installation, now you need Client ID and Client secret which can be looked up at Aspose Cloud Dashboard. Therefore, you need to first sign up for an account on our Dashboard and retrieve your credentials.
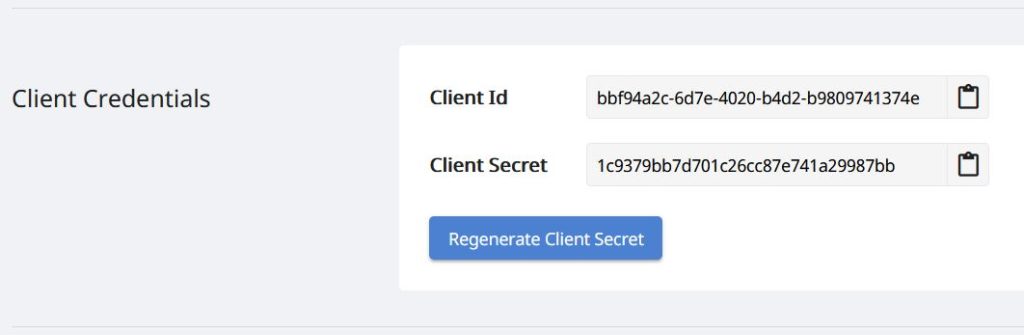
Image 1:- Client Credentials on Cloud dashboard.
JPG to Word Conversion using Python
In order to convert JPG to Word using Python SDK, we need to first place an individual image on each page of the PDF document and then convert the complete PDF file to DOC format. Please follow the instructions specified below.
- Firstly, initialize an object of ApiClient while passing Client credentials as arguments
- Secondly, create an object of PdfApi which takes the ApiClient object as an input argument
- Thirdly, specify the names of JPG images to be placed inside the document, PDF file name
- Now call the put_create_document(…) method to create a blank PDF in cloud storage
- The next step is to insert a JPG image on the first page of the newly created PDF file using the post_insert_image(…) method
- Call the method put_add_new_page(…) to insert a new blank page at the end of the PDF document
- Again call the post_insert_image(…) method to place another image on the 2nd-page pdf PDF file
- Finally, call the method put_pdf_in_storage_to_doc(…) to render the PDF file to DOC format and save the output in cloud storage
JPG to DOC Converter using cURL Command
Owing to the REST architecture of Aspose.PDF Cloud, it can also be accessed via cURL commands. However, in order to ensure data privacy and integrity, only authorized users can access our Cloud services. So based on client credentials generated earlier, we need to first generate a JWT access token to authenticate and access cloud services. Please execute the following command to generate the JWT token.
curl -v "https://api.aspose.cloud/connect/token" \
-X POST \
-d "grant_type=client_credentials&client_id=bbf94a2c-6d7e-4020-b4d2-b9809741374e&client_secret=1c9379bb7d701c26cc87e741a29987bb" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "Accept: application/json"
Once the token has been generated, we need to execute the following command to generate a blank PDF file in cloud storage.
curl -v -X PUT "https://api.aspose.cloud/v3.0/pdf/Source.pdf" \
-H "accept: application/json" \
-H "authorization: Bearer <JWT Token>"
Now use the following command to insert a JPG image already available in cloud storage to the first page of the document.
curl -X POST "https://api.aspose.cloud/v3.0/pdf/Source.pdf/pages/2/images?llx=10&lly=10&urx=10&ury=10" \
-H "accept: application/json" \
-H "authorization: Bearer <JWT Token>" \
-H "Content-Type: multipart/form-data" \
-d {"image":{}}
In order to add a new image to the PDF document, please insert a blank page at the end of the existing PDF file using the following cURL command
curl -X PUT "https://api.aspose.cloud/v3.0/pdf/Source.pdf/pages" \
-H "accept: application/json" \
-H "authorization: Bearer <JWT Token>"
Again call the earlier specified cURL command to add an image to the second page of the PDF file and then call the following command to convert the PDF to MS Word format. In the following command, we have specified the output file format as DOC. We know that the API allows us to control how a PDF document is converted into a word processing document, so we have specified the mode value as Flow.
curl -X PUT "https://api.aspose.cloud/v3.0/pdf/source.pdf/convert/doc?outPath=Resultant.doc&format=Doc&mode=Flow" \
-H "accept: application/json" \
-H "authorization: Bearer <JWT Token>"
Conclusion
In this blog, we have discussed the details on how to develop a JPG to Word converter using Python SDK. Similarly, we have also explored the details on how to use the cURL commands to implement JPG to DOC converter in the command line terminal. In case you have a requirement to save Word to JPG, please try using Aspose.Words Cloud SDK for Python. For further information, please visit Convert Documents using Python.
Now coming back to Aspose.PDF Cloud SDK for Python, its complete source code can be downloaded from GitHub. We also recommend you explore the Developer Guide to learn more about other exciting features being offered by Cloud API.
Related Articles
We recommend you to visit the following links to learn more about