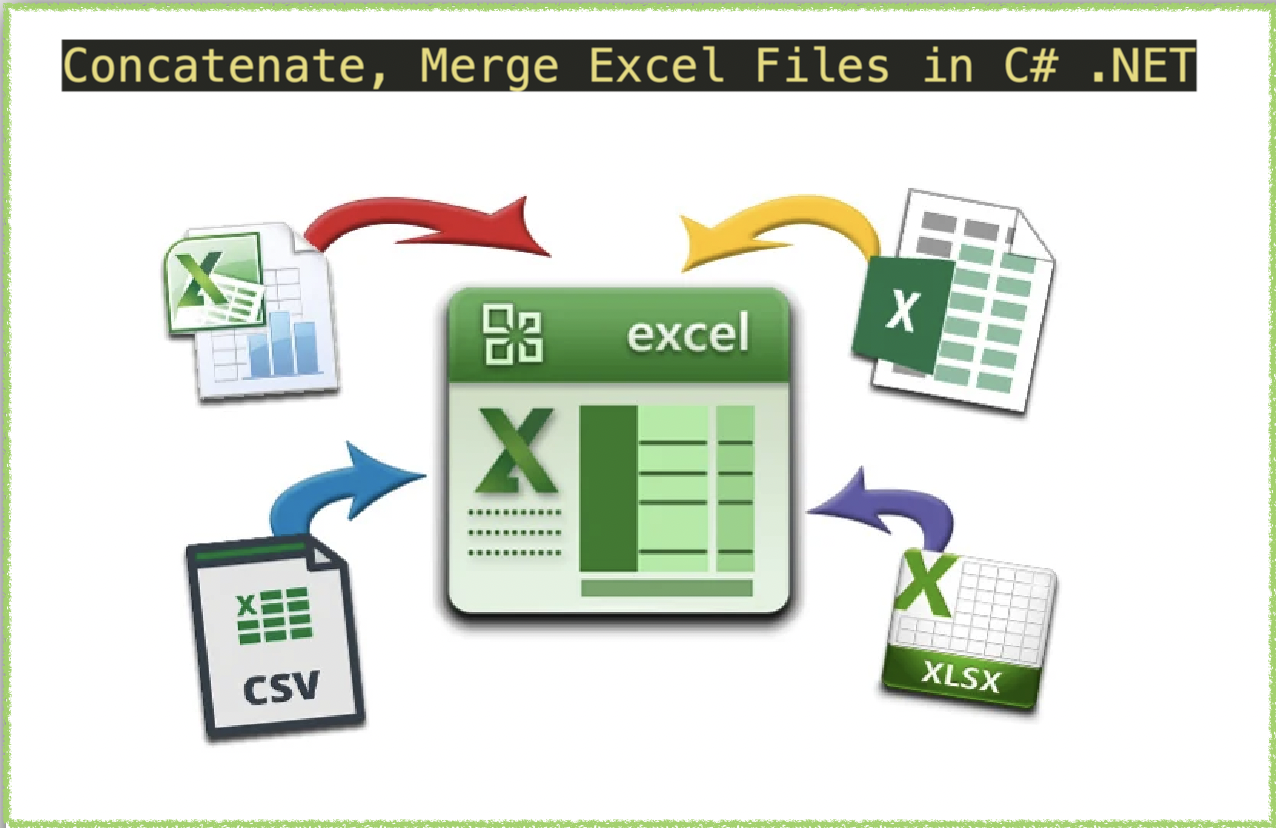
Concatenate Excel (XLS, XLSX) files in C# .NET
Combining Excel files can be a useful technique for streamlining data management. Whether you need to consolidate sales reports, financial statements, or customer data, merging Excel files can save you time and effort. In this article, we will explore how to concatenate Excel files using C# .NET and REST API. We will cover different scenarios where concatenation can be useful, such as when you have multiple files with similar data structures, or when you need to merge data from different formats. You will learn how to write simple and efficient code to automate the concatenation process and avoid manual errors. So, let’s dive into the world of Excel concatenation and simplify your workflow today.
Concatenate Excel REST API
If you’re looking for an efficient and straightforward way to concatenate Excel files using C# .NET, then Aspose.Cells Cloud SDK is a great option. It offers a simple interface to merge XLS, XLSX, and other file formats using REST APIs. By using this cloud-based solution, you can eliminate the need for installing complex software and hardware infrastructure. The Aspose.Cells Cloud SDK for .NET also provides features like auto-fitting rows and columns, sorting data, and applying formatting to merged cells. By using this API, you can significantly reduce development time and effort and improve productivity.
Now, in order to use the SDK, we are going to add its reference in our application through NuGet package manager. Simply search “Aspose.Cells-Cloud” and hit the Add Package button. Secondly, if you do not have an account over Cloud Dashboard, please create a free account using a valid email address and obtain your personalized client credentials.
Merge Excel using C#
In this section, we are going to load two worksheets from cloud storage and then merge the second Excel workbook to the first.
Let’s develop our understanding about above code snippet:
CellsApi cellsInstance = new CellsApi(clientID, clientSecret);
Create an object of CellsApi while passing client credentials as arguments.
Dictionary<String, Stream> mapFiles = new Dictionary<string, Stream>();
Create Dictionary object which will hold names and content of input Excel workbooks.
mapFiles.Add(first_Excel, File.OpenRead(first_Excel));
Add input Excel files to dictionary object. We are adding files in key-value pairs.
foreach (KeyValuePair<String, Stream> dictionary in mapFiles)
{
// upload each workbook to cloud storage
cellsInstance.UploadFile(dictionary.Key, dictionary.Value);
}
Iterate through dictionary instance and upload each Excel workbook to cloud storage.
// initialize the conversion operation
var response = cellsInstance.CellsWorkbookPostWorkbooksMerge(first_Excel, second_Excel, folder: null, storageName: null, mergedStorageName: null);
Call the method to initiate the Excel merge operation. All the worksheets from second Excel workbook are merged into first Excel workbook.
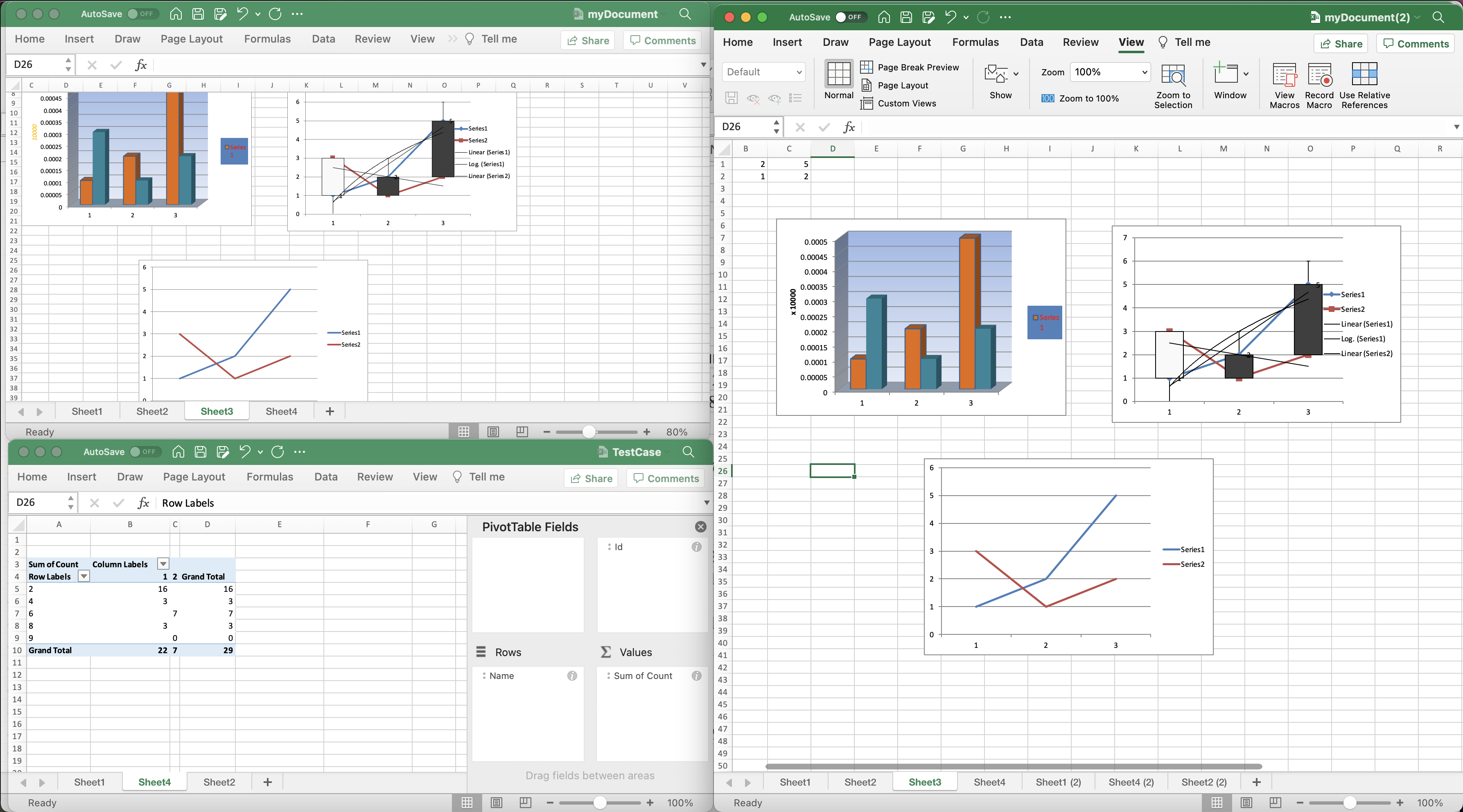
Combined Excel workbooks preview.
The sample Excel workbooks used in the above example can be downloaded from myDocument.xlsx and TestCase.xlsx respectively.
Combine Excel using cURL Commands
Combining Excel files using REST API is an excellent approach. The REST APIs provide a simple and efficient way to merge Excel files and can be easily integrated with other software tools. One of the major strengths of using REST API is the ability to work with different file formats, including XLS, XLSX, CSV, and more. Additionally, REST APIs are highly scalable and can handle large data sets, making them an excellent choice for enterprise-level data management. When combining Excel workbooks using REST APIs, you can save development time and efforts, improve data accuracy, and simplify your workflow.
Now, first we need to generate a JWT access token based on client credentials:
curl -v "https://api.aspose.cloud/connect/token" \
-X POST \
-d "grant_type=client_credentials&client_id=bb959721-5780-4be6-be35-ff5c3a6aa4a2&client_secret=4d84d5f6584160cbd91dba1fe145db14" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "Accept: application/json"
Once we have JWT token, we need to use PostWorkbooksMerge API to combine Excel workbooks. The merged Excel will remain in Cloud storage.
curl -v -X POST "https://api.aspose.cloud/v3.0/cells/TestCase.xlsx/merge?mergeWith=myDocument(1).xlsx" \
-H "accept: application/json" \
-H "authorization: Bearer <JWT Token>"
Concluding Remarks
In conclusion, combining Excel files using C# .NET and REST APIs is an effective way to streamline your data management process and increase productivity. Whether you need to consolidate data from multiple sources (XLS, XLSX etc.) or automate repetitive tasks, concatenating Excel files can save you time and effort. By leveraging the power of cloud-based REST APIs, you can perform data manipulation tasks without the need for complex software installations or hardware infrastructure. We have also learned that CURL commands can also be used to test and integrate REST APIs with other software tools. So, whether you’re a beginner or an experienced developer, merging Excel files using REST API and CURL commands is an approach worth considering. Lastly, by following the simple steps outlined in this article, you can start merging Excel files with ease and streamline your workflow.
In case you encounter any issues while using the API, please contact us via customer support forum.
Related Articles
Please visit the following links to learn more about: