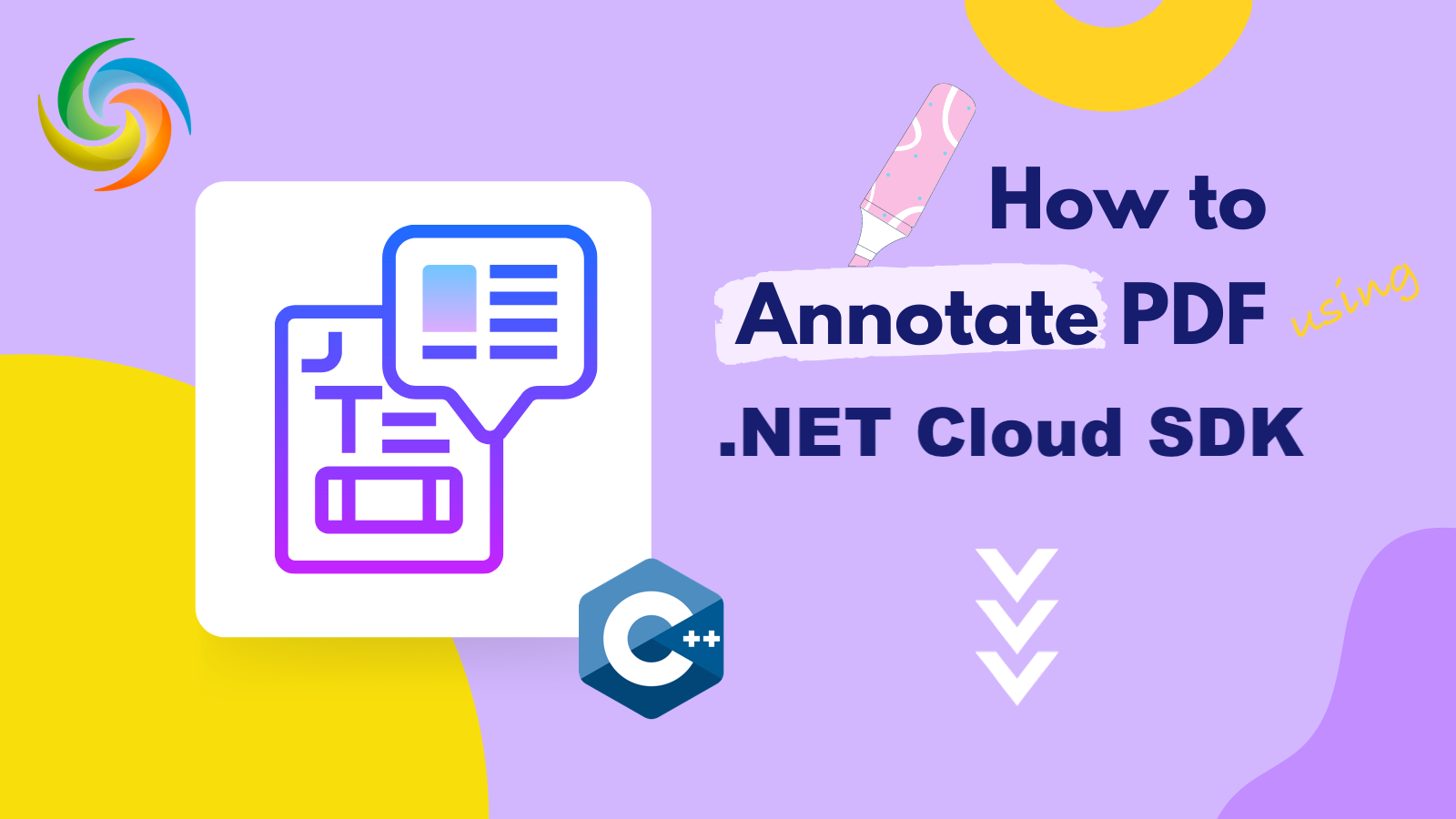
How to add annotations to a PDF using C# .NET
In today’s digital age, PDFs have become a popular format for sharing information, documents, and reports. However, simply viewing a PDF may not be enough for many users. Sometimes, users may want to highlight or add notes to specific parts of the PDF to provide additional context or feedback. Fortunately, there are several free PDF annotators available that allow users to easily add comments, highlight text, and more. However, in this article, we will explore how to use the REST API to support PDF annotations, and show you how to highlight, comment, and add notes to your PDF documents.
- REST API for PDF Annotation
- Add Comments to PDF using C#
- Add Free Text Annotation to PDF using cURL Commands
REST API for PDF Annotation
Aspose.PDF Cloud provides an easy-to-use and comprehensive solution to add annotations to PDF documents programmatically. With Aspose.PDF Cloud, you can add various types of annotations to PDF documents, including text, image, stamp, and various markup annotations. You can also modify existing annotations, such as changing the position, size, color, or any other properties.
Supported Annotations include Text, Circle, Polygon, PolyLine, Line, Square, FreeText, Highlight, Underline, Squiggly, StrikeOut, Caret, Ink, Link, Popup, FileAttachment, Sound, Movie, Screen, Widget, Watermark, TrapNet, PrinterMark, Redaction, Stamp, RichMedia and PDF3D.
Now, in order to add the SDK in your project, please search Aspose.PDF-Cloud
in NuGet packages manager and click the Add Package button. The next important step is to register an account over Cloud dashboard and get your personal client credentials. Please take a look over the Quick Start guide for further details.
Add Comments to PDF using C#
Let’s have a look over the C# .NET code snippet which is used to add Annotation to a PDF document.
Now, lets understand the code snippet in more details.
PdfApi api = new PdfApi(clientSecret, clientID);
Create an instance of PdfApi class which takes client credentials as arguments in its constructor.
List<FreeTextAnnotation> annotations = new List<FreeTextAnnotation>
As the API supports the capability to add one or more Annotations of similar type at the same time, so we need to create a List object of FreeTextAnnnotation type.
new FreeTextAnnotation(
Rect: new Rectangle(100, 800, 350, 830),
TextStyle:new TextStyle(
FontSize: 26, Font: "Arial",
ForegroundColor: new Aspose.Pdf.Cloud.Sdk.Model.Color(0xFF, 0, 0xFF, 0),
BackgroundColor: new Aspose.Pdf.Cloud.Sdk.Model.Color(0xFF, 0xFF, 0, 0)
))
Create an object of FreeTextAnnotation where we define the rectangular region for Annotation and text formatting details.
Contents = "Confidential !"
Here we define the content for FreeTextAnnotation.
var response = api. PostPageFreeTextAnnotations("Binder1.pdf", 1, annotations);
Call the REST API to add FreeTextAnnotation on the first page of PDF document already available in Cloud storage.
Given below are the possible values that can be assigned to the properties used in above code snippet.
- AnnotationFlags - Supported values can be
[Default, Invisible, Hidden, Print, NoZoom, NoRotate, NoView, ReadOnly, Locked, ToggleNoView, LockedContents]
.- Rotate - Rotation angle for text. Possible values can be
[None, on90, on180, on270]
.- AnnotationFlags - A set of flags specifying various characteristics of the annotation. Possible value can be
[Default, Invisible, Hidden, Print, NoZoom, NoRotate, NoView, ReadOnly, Locked, ToggleNoView, LockedContents]
.- FreeTextIntent - Enumerates the intents of the free text annotation. Possible values can be
[ Undefined, FreeTextCallout, FreeTextTypeWriter]
.
Add Free Text Annotation to PDF using cURL Commands
The usage of cURL commands to call Aspose.PDF Cloud API is a good approach to accomplish this requirement. It is also good if you are familiar with command-line tools or prefer to use them. So, with the cURL command-line tool, you can make HTTP requests and perform various operations regarding PDF file processing.
Now, in order to add annotations to a PDF document using cURL commands, first we need to generate an authentication token by sending a request to the token endpoint with your App SID and App Key. Please execute the following command to generate the accessToken.
curl -v "https://api.aspose.cloud/connect/token" \
-X POST \
-d "grant_type=client_credentials&client_id=ee170169-ca49-49a4-87b7-0e2ff815ea6e&client_secret=7f098199230fc5f2175d494d48f2077c" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "Accept: application/json"
Once the accessToken is generated, please execute the following command which adds the FreeTextAnnotation to the PDF document. The Annotated PDF file is then updated on Cloud storage.
curl -v "https://api.aspose.cloud/v3.0/pdf/{inputPDF}/pages/1/annotations/freetext" \
-X POST \
-H "accept: application/json" \
-H "authorization: Bearer {accessToken}" \
-H "Content-Type: application/json" \
-d "[ { \"Color\": { \"A\": 0, \"R\": 0, \"G\": 0, \"B\": 0 }, \"Contents\": \"Confidential !\", \"Modified\": \"01/05/2023 12:00:00.000 PM\", \"Id\": \"id0\", \"Flags\": [ \"Default\" ], \"Name\": \"comment\", \"Rect\": { \"LLX\": 100, \"LLY\": 800, \"URX\": 350, \"URY\": 830 }, \"PageIndex\": 0, \"ZIndex\": 1, \"HorizontalAlignment\": \"Center\", \"VerticalAlignment\": \"Center\", \"CreationDate\": \"03/05/2023 16:00:00.000 PM\", \"Subject\": \"Subj.\", \"Title\": \"Main Heading\", \"RichText\": \"Hello world...\", \"Justification\": \"Left\", \"Intent\": \"FreeTextTypeWriter\", \"Rotate\": \"None\", \"TextStyle\": { \"FontSize\": 26, \"Font\": \"Arial\", \"ForegroundColor\": { \"A\": 10, \"R\": 10, \"G\": 100, \"B\": 120 }, \"BackgroundColor\": { \"A\": 0, \"R\": 0, \"G\": 50, \"B\": 80 } } }]"
Replace
{inputPDF}
with the name of input PDF file already available on Cloud storage,{accessToken}
with JWT access token generated above.
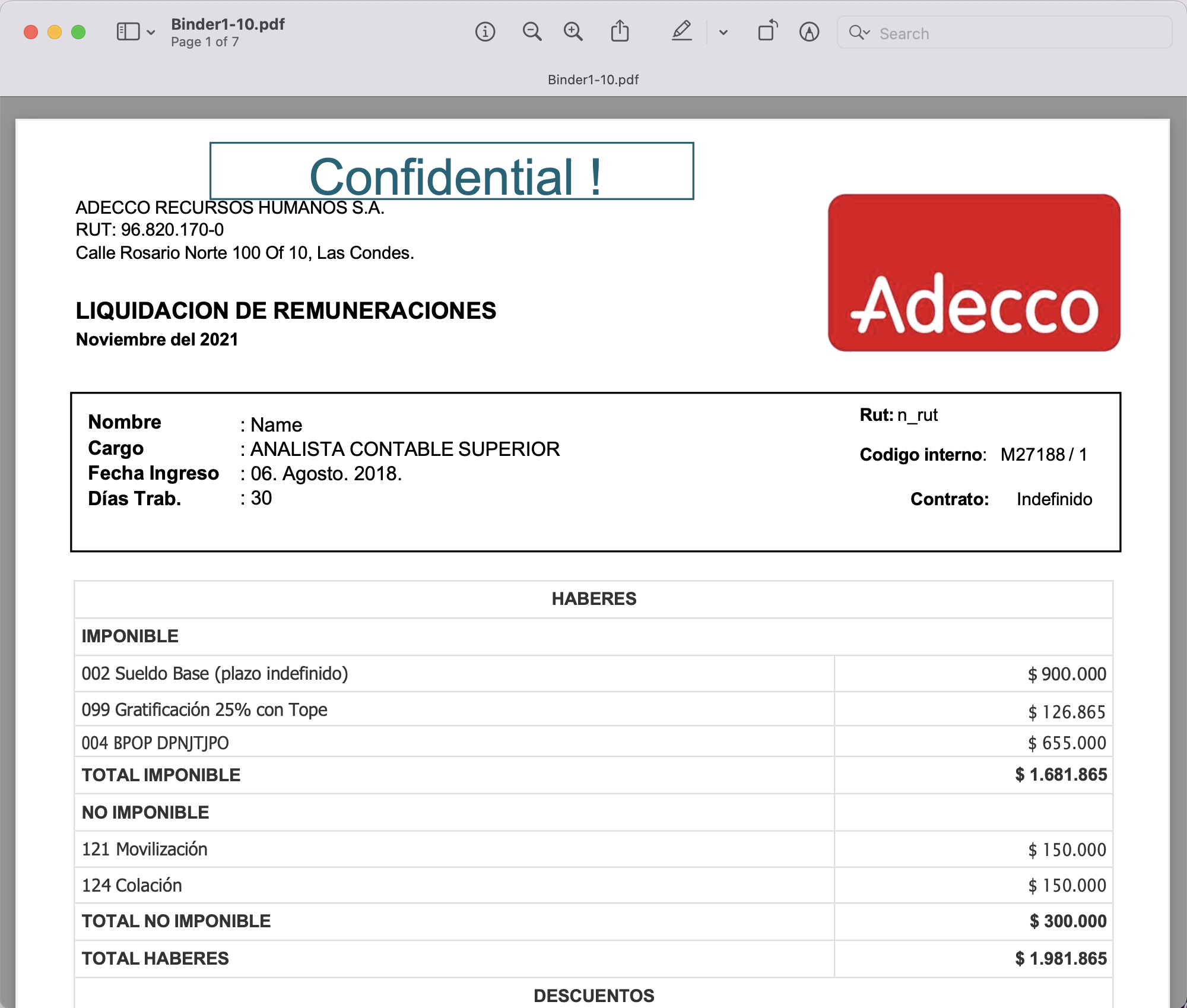
Image:- Preview of Free Text Annotation in PDF file.
The PDF document used in the above example can be downloaded from this link.
Conclusion
In conclusion, adding annotations to PDFs can greatly enhance their usefulness and functionality. Whether you are looking to highlight important text, add comments, or take notes directly within the document, then Aspose.PDF Cloud is an amazing choice to achieve this. We have also learnt that Aspose.PDF Cloud SDK for .NET and cURL commands offer powerful solutions for annotating PDFs, giving you the ability to create customized workflows and automation processes. Therefore, with these tools, you can quickly and easily add annotations to PDFs, while saving time and increasing productivity.
Useful Links
Related Articles
We highly recommend going through the following blogs: