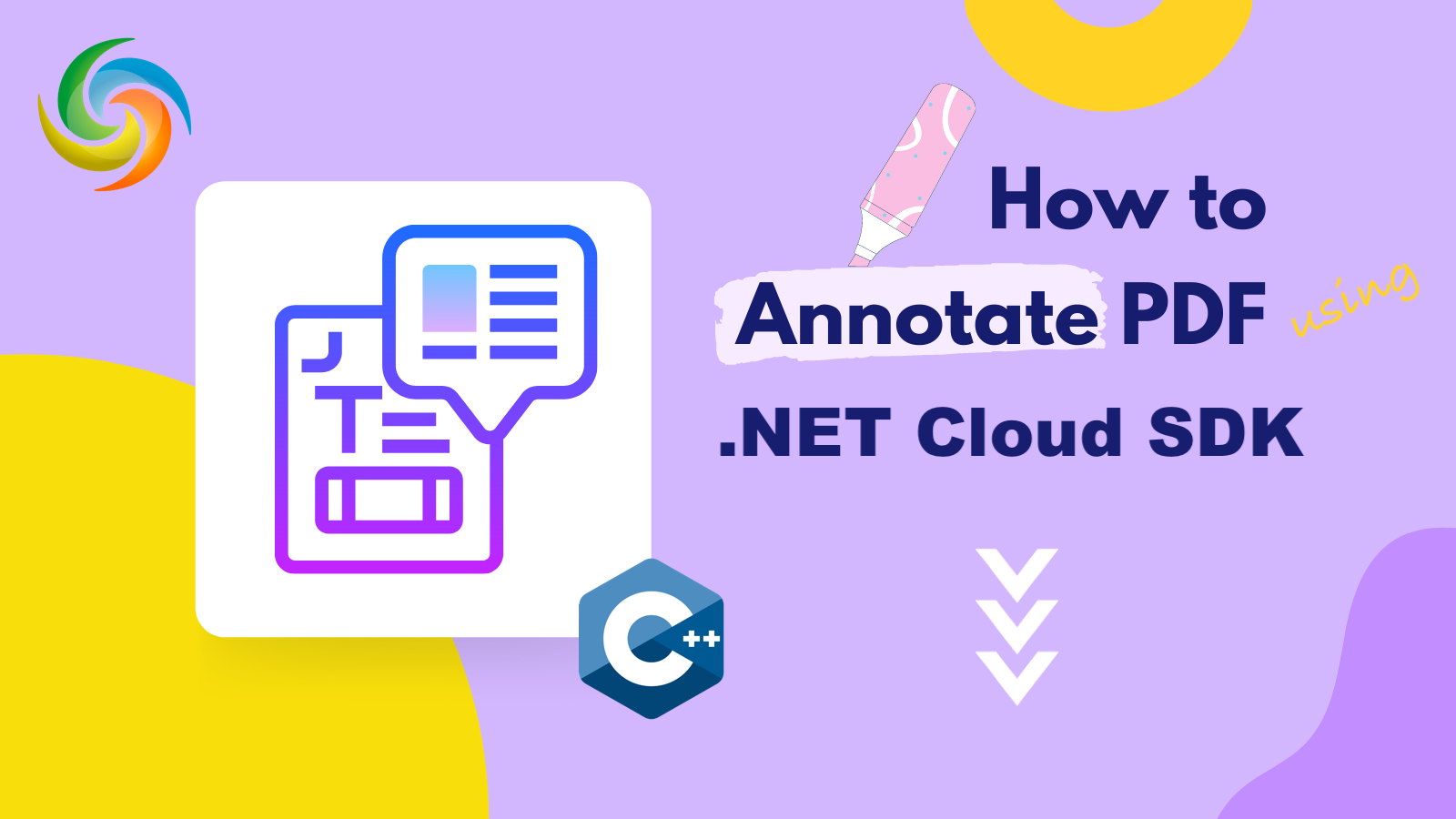
如何使用 C# .NET 向 PDF 添加注释
在当今的数字时代,PDF 已成为共享信息、文档和报告的流行格式。但是,对于许多用户来说,仅仅查看 PDF 可能还不够。有时,用户可能希望突出显示或向 PDF 的特定部分添加注释以提供额外的上下文或反馈。幸运的是,有几个免费的 PDF 注释器可以让用户轻松添加注释、突出显示文本等。然而,在本文中,我们将探讨如何使用 REST API 来支持 PDF 注释,并向您展示如何为 PDF 文档高亮、注释和添加注释。
用于 PDF 注释的 REST API
Aspose.PDF Cloud 提供了一个易于使用且全面的解决方案,以编程方式向 PDF 文档添加注释。使用Aspose.PDF Cloud,您可以为PDF文档添加各种类型的注释,包括文本、图像、图章和各种标记注释。您还可以修改现有注释,例如更改位置、大小、颜色或任何其他属性。
支持的注释包括文本、圆形、多边形、折线、直线、正方形、自由文本、突出显示、下划线、波浪形、删除线、插入符号、墨迹、链接、弹出窗口、文件附件、声音、电影、屏幕、小部件、水印、TrapNet、PrinterMark、 Redaction、Stamp、RichMedia 和 PDF3D。
现在,为了在您的项目中添加 SDK,请在 NuGet 包管理器中搜索“Aspose.PDF-Cloud”并单击“添加包”按钮。下一个重要步骤是通过云仪表板注册一个帐户并获取您的个人客户凭据。请查看快速入门 指南以了解更多详细信息。
使用 C# 向 PDF 添加注释
让我们看一下用于向 PDF 文档添加注释的 C# .NET 代码片段。
// 如需完整示例和数据文件,请访问
https://github.com/aspose-pdf-cloud/aspose-pdf-cloud-dotnet
// 从 https://dashboard.aspose.cloud/ 获取客户端凭证
string clientSecret = "7f098199230fc5f2175d494d48f2077c";
string clientID = "ee170169-ca49-49a4-87b7-0e2ff815ea6e";
// 创建 PdfApi 对象
PdfApi api = new PdfApi(clientSecret, clientID);
// 创建包含 FreeTextAnnotations 的列表对象
List<FreeTextAnnotation> annotations = new List<FreeTextAnnotation>
{
new FreeTextAnnotation(
// 指定包含 FreeTextAnnotation 的矩形区域
// 还定义文本格式详细信息
Rect: new Rectangle(100, 800, 350, 830),
TextStyle:new TextStyle(
FontSize: 26, Font: "Arial",
ForegroundColor: new Aspose.Pdf.Cloud.Sdk.Model.Color(0xFF, 0, 0xFF, 0),
BackgroundColor: new Aspose.Pdf.Cloud.Sdk.Model.Color(0xFF, 0xFF, 0, 0)
))
{
// FreeTextAnnotation 中要显示的内容
Contents = "Confidential !",
Color = new Aspose.Pdf.Cloud.Sdk.Model.Color(0, 0, 0, 0),
Id = "id1",
Name = "Test Free Text",
Flags = new List<AnnotationFlags> {AnnotationFlags.Default},
HorizontalAlignment = HorizontalAlignment.Center,
Intent = FreeTextIntent.FreeTextTypeWriter,
RichText = "Rich Text",
Subject = "Text Box Subj",
ZIndex = 1,
Justification = Justification.Center,
Title = "Title",
PageIndex = 1
}
};
var response = api. PostPageFreeTextAnnotations("Binder1.pdf", 1, annotations);
现在,让我们更详细地了解代码片段。
PdfApi api = new PdfApi(clientSecret, clientID);
创建一个 PdfApi 类的实例,该实例将客户端凭据作为其构造函数中的参数。
List<FreeTextAnnotation> annotations = new List<FreeTextAnnotation>
由于 API 支持同时添加一个或多个相似类型的 Annotation,因此我们需要创建一个 FreeTextAnnnotation 类型的 List 对象。
new FreeTextAnnotation(
Rect: new Rectangle(100, 800, 350, 830),
TextStyle:new TextStyle(
FontSize: 26, Font: "Arial",
ForegroundColor: new Aspose.Pdf.Cloud.Sdk.Model.Color(0xFF, 0, 0xFF, 0),
BackgroundColor: new Aspose.Pdf.Cloud.Sdk.Model.Color(0xFF, 0xFF, 0, 0)
))
创建一个 FreeTextAnnotation 对象,我们在其中定义注释和文本格式详细信息的矩形区域。
Contents = "Confidential !"
这里我们定义了 FreeTextAnnotation 的内容。
var response = api. PostPageFreeTextAnnotations("Binder1.pdf", 1, annotations);
调用 REST API 在云存储中已有的 PDF 文档的第一页上添加 FreeTextAnnotation。
下面给出了可以分配给上述代码片段中使用的属性的可能值。
- AnnotationFlags - 支持的值可以是
[Default, Invisible, Hidden, Print, NoZoom, NoRotate, NoView, ReadOnly, Locked, ToggleNoView, LockedContents]
。- 旋转 - 文本的旋转角度。可能的值可以是“[None, on90, on180, on270]”。
- AnnotationFlags - 一组指定注释的各种特征的标志。可能的值可以是“[Default, Invisible, Hidden, Print, NoZoom, NoRotate, NoView, ReadOnly, Locked, ToggleNoView, LockedContents]”。
- FreeTextIntent - 枚举自由文本注释的意图。可能的值可以是“[未定义、FreeTextCallout、FreeTextTypeWriter]”。
使用 cURL 命令向 PDF 添加自由文本注释
使用 cURL 命令调用 Aspose.PDF Cloud API 是完成此要求的好方法。如果您熟悉命令行工具或更喜欢使用它们,那也很好。因此,使用 cURL 命令行工具,您可以发出 HTTP 请求并执行有关 PDF 文件处理的各种操作。
现在,为了使用 cURL 命令向 PDF 文档添加注释,首先我们需要通过使用您的 App SID 和 App Key 向令牌端点发送请求来生成身份验证令牌。请执行以下命令生成accessToken。
curl -v "https://api.aspose.cloud/connect/token" \
-X POST \
-d "grant_type=client_credentials&client_id=ee170169-ca49-49a4-87b7-0e2ff815ea6e&client_secret=7f098199230fc5f2175d494d48f2077c" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "Accept: application/json"
生成 accessToken 后,请执行以下命令,将 FreeTextAnnotation 添加到 PDF 文档。然后在云存储上更新带注释的 PDF 文件。
curl -v "https://api.aspose.cloud/v3.0/pdf/{inputPDF}/pages/1/annotations/freetext" \
-X POST \
-H "accept: application/json" \
-H "authorization: Bearer {accessToken}" \
-H "Content-Type: application/json" \
-d "[ { \"Color\": { \"A\": 0, \"R\": 0, \"G\": 0, \"B\": 0 }, \"Contents\": \"Confidential !\", \"Modified\": \"01/05/2023 12:00:00.000 PM\", \"Id\": \"id0\", \"Flags\": [ \"Default\" ], \"Name\": \"comment\", \"Rect\": { \"LLX\": 100, \"LLY\": 800, \"URX\": 350, \"URY\": 830 }, \"PageIndex\": 0, \"ZIndex\": 1, \"HorizontalAlignment\": \"Center\", \"VerticalAlignment\": \"Center\", \"CreationDate\": \"03/05/2023 16:00:00.000 PM\", \"Subject\": \"Subj.\", \"Title\": \"Main Heading\", \"RichText\": \"Hello world...\", \"Justification\": \"Left\", \"Intent\": \"FreeTextTypeWriter\", \"Rotate\": \"None\", \"TextStyle\": { \"FontSize\": 26, \"Font\": \"Arial\", \"ForegroundColor\": { \"A\": 10, \"R\": 10, \"G\": 100, \"B\": 120 }, \"BackgroundColor\": { \"A\": 0, \"R\": 0, \"G\": 50, \"B\": 80 } } }]"
将
{inputPDF}
替换为云存储上已有的输入 PDF 文件的名称,将{accessToken}
替换为上面生成的 JWT 访问令牌。
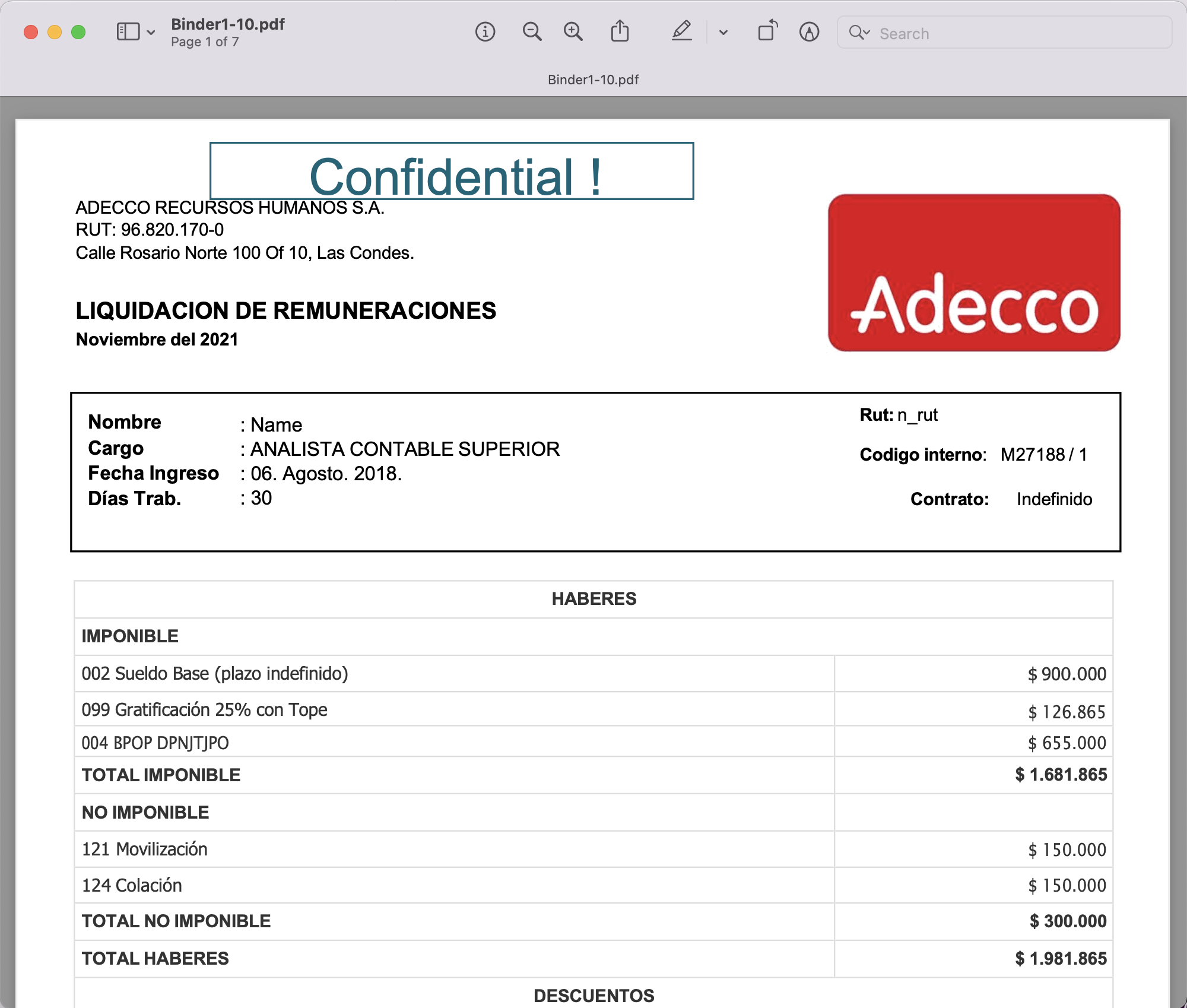
图像:- PDF 文件中自由文本注释的预览。
上例中使用的PDF文档可以从此链接下载。
结论
总之,向 PDF 添加注释可以极大地增强其实用性和功能。无论您是要突出显示重要文本、添加评论还是直接在文档中做笔记,Aspose.PDF Cloud 都是实现这一目标的绝佳选择。我们还了解到,Aspose.PDF Cloud SDK for .NET 和 cURL 命令为注释 PDF 提供了强大的解决方案,使您能够创建自定义工作流和自动化流程。因此,使用这些工具,您可以快速轻松地向 PDF 添加注释,同时节省时间并提高工作效率。
有用的链接
相关文章
我们强烈建议浏览以下博客: