Java SDK を使用してバーコード スキャナー アプリを開発する方法。 Web、モバイル、およびデスクトップ アプリケーションに QR スキャン機能を実装します。
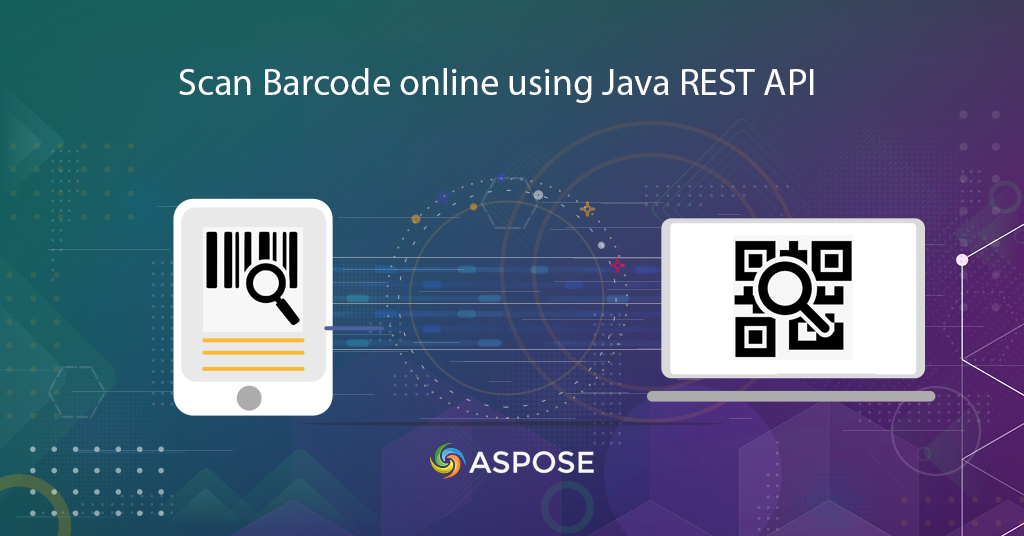
バーコードをオンラインでスキャン | QRコードスキャナー
この記事では、Java REST API を使用してバーコード スキャナーと QR コード スキャナー アプリを開発する方法の詳細について説明します。今日のペースの速い経済において、バーコードはベンダーやマーチャントが製品の詳細を保存するための不可欠で実行可能なソリューションであることを理解しています。長年にわたり、それらは企業にとって価値があり実行可能な選択肢であることが証明されています。効率が大幅に向上し、オーバーヘッドが削減されました。バーコードは、費用対効果が高く、信頼性があります。 BarCode を使用するその他の利点の中で、以下に指定するその他の利点をいくつか示します。
- バーコードは人的エラーの可能性を排除します
- バーコード システムを使用すると、従業員のトレーニング時間が短縮されます
- バーコードは非常に汎用性が高く、あらゆる種類の必要なデータ収集に使用できます
- 正確な在庫管理が可能になり、在庫管理が向上します
- さらに、バーコードはより良いデータを提供します。つまり、単一のバーコードで在庫と価格の詳細を提供できます。
このようなすべての機能に関連する Aspose.BarCode Cloud Java SDK は、Java 開発者が Java 言語を使用してオンラインでバーコードを作成およびスキャンできるようにします。他の Cloud API と同様に、Aspose.BarCode Cloud Java SDK では、[Cloud Dashboard] でアカウントを登録する必要があります。2 既にアカウントを登録している場合は、引き続き使用できます。アカウントの準備ができたら、AppKey と AppSID を介してクラウド サービスを使用できます。
Aspose クラウド ストレージの使用を検討するか、ファイルの保存と取得にサードパーティのクラウド ストレージ サービスを使用することを検討してください。
サポートされているバーコード記号
SDK は、EAN、UPC、Codabar、PDF417、QR、MicroQR、Postnet、Planet、RM4SCC など、多数のバーコード記号 (60 以上) をサポートしています。既存のバーコード情報を読み込んで、出力を一般的な画像に保存するオプションもあります。 JPEG、PNG、GIF、BMP、TIFF、EMF、WMF、SVG、EXIF、および ICON。サポートされているシンボル体系の完全なリストについては、Aspose.BarCode Cloud Java SDK を参照してください。
バーコードを生成
SDK を使用すると、線形、2D、および郵便のバーコード イメージをさまざまな形式で作成できます。画像の幅、高さ、境界線のスタイル、出力画像形式など、バーコード画像の属性を指定できます。また、アプリケーションの要件に応じて、バーコード タイプと、テキストの位置やフォント スタイルなどのテキスト属性を指定することもできます。また、バーの高さを設定し、バーコード画像をある角度で回転させる機能も提供します。
次の例は、Code39Standard バーコードを作成し、ページの上中央揃えに配置する手順を示しています。テキストの色はネイビー、水平方向、垂直方向の解像度は 200 に指定されています。BarColor はオレンジ、背景色はシルバー、出力形式は JPEG 形式に指定されています。
先に進む前に、cURL コマンドを使用して API にアクセスする際に JWT トークンが必要になるため、次のリンクにアクセスすることをお勧めします。
カール
curl -X PUT "https://api.aspose.cloud/v3.0/barcode/MySample.jpeg/generate?Type=Code39Standard&Text=BarCode%20processing&TextLocation=Above&TextAlignment=Center&TextColor=Navy&FontSizeMode=Auto&Resolution=200&ResolutionX=200&BackColor=silver&BarColor=Orange&BorderColor=Blue&format=jpeg" \
-H "accept: application/json" \
-H "authorization: Bearer <JWT Token>"
リクエスト URL
https://api.aspose.cloud/v3.0/barcode/MySample.jpeg/generate?Type=Code39Standard&Text=BarCode%20processing&TextLocation=Above&TextAlignment=Center&TextColor=Navy&FontSizeMode=Auto&Resolution=200&ResolutionX=200&BackColor=silver&BarColor=Orange&BorderColor=Blue&format=jpeg
ジャワ
ApiClient client = new ApiClient(
"App SID from https://dashboard.aspose.cloud/#/apps",
"App Key from https://dashboard.aspose.cloud/#/apps");
com.aspose.barcode.cloud.api.BarcodeApi api = new com.aspose.barcode.cloud.api.BarcodeApi(client);
String name = "MySample.jpeg";
String type = com.aspose.barcode.cloud.model.EncodeBarcodeType.CODE39STANDARD.getValue();
String text = "Barcode processing API"; // String | Text to encode.
String twoDDisplayText = null;
String textLocation = com.aspose.barcode.cloud.model.CodeLocation.ABOVE.getValue();
String textAlignment = com.aspose.barcode.cloud.model.TextAlignment.CENTER.getValue();
String textColor = "Navy";
String fontSizeMode = com.aspose.barcode.cloud.model.FontMode.AUTO.getValue();
int resolution = 200;
double resolutionX = 200;
double resolutionY = 200;
String barColor = "Orange";
String format = "JPEG";
try {
com.aspose.barcode.cloud.model.ResultImageInfo result = api.putBarcodeGenerateFile(
name,
type,
text,
twoDDisplayText,
textLocation,
textAlignment,
textColor,
fontSizeMode,
(double) resolution,
resolutionX,
resolutionY,
null,
null,
null,
null,
null,
null,
null,
null,
"Silver",
barColor,
"Blue",
null,
null,
true,
null,
null,
null,
null,
null,
null,
null,
null,
null,
null,
format);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling BarcodeApi#PutBarcodeGenerateFile");
e.printStackTrace();
}
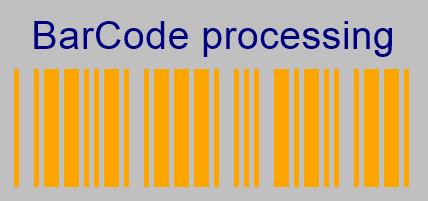
画像 1:- 結果のバーコード プレビュー。
バーコード リーダー オンライン
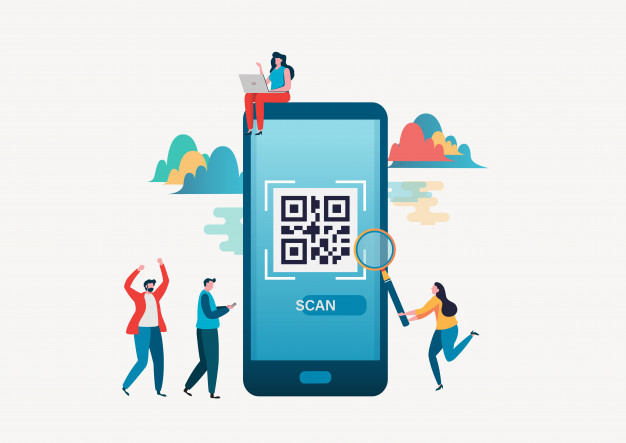
画像 2: QR コード スキャナー
Cloud API は、既存のバーコードからの情報を認識することもできます。すばやく取得できるようにバーコード タイプの詳細を指定するか、API にタイプを自動的に判別させるオプションがあります。 ChecksumValidation の詳細、DetectEncoding を指定することも、実行時に API に決定させることもできます。
カール
curl -X POST "https://api.aspose.cloud/v3.0/barcode/recognize?Type=all&DetectEncoding=true" \
-H "accept: application/json" \
-H "authorization: Bearer <JWT Token>" \
-H "Content-Type: multipart/form-data" \
-H "x-aspose-client: Containerize.Swagger" -d {"image":{}}
リクエスト URL
https://api.aspose.cloud/v3.0/barcode/recognize?Type=all&DetectEncoding=true
ジャワ
// 完全な例とデータ ファイルについては、https://github.com/aspose-barcode-cloud/aspose-barcode-cloud-java/ にアクセスしてください。
String type = null;
String checksumValidation = ChecksumValidation.OFF.toString();
Boolean detectEncoding = null;
String preset = PresetType.HIGHPERFORMANCE.toString();
Integer rectX = null;
Integer rectY = null;
Integer rectWidth = null;
Integer rectHeight = null;
Boolean stripFNC = null;
Integer timeout = null;
Integer medianSmoothingWindowSize = null;
Boolean allowMedianSmoothing = null;
Boolean allowComplexBackground = null;
Boolean allowDatamatrixIndustrialBarcodes = null;
Boolean allowDecreasedImage = null;
Boolean allowDetectScanGap = null;
Boolean allowIncorrectBarcodes = null;
Boolean allowInvertImage = null;
Boolean allowMicroWhiteSpotsRemoving = null;
Boolean allowOneDFastBarcodesDetector = null;
Boolean allowOneDWipedBarsRestoration = null;
Boolean allowQRMicroQrRestoration = null;
Boolean allowRegularImage = null;
Boolean allowSaltAndPepperFiltering = null;
Boolean allowWhiteSpotsRemoving = null;
Double regionLikelihoodThresholdPercent = null;
List<Integer> scanWindowSizes = null;
Double similarity = null;
Boolean skipDiagonalSearch = null;
String australianPostEncodingTable = null;
String rectangleRegion = null;
String url = null;
Path currentRelativePath = Paths.get("");
String currentPath = currentRelativePath.toAbsolutePath().toString();
Path filePath = Paths.get(currentPath, "data", "sample.png");
File image = new File(String.valueOf(filePath));
BarcodeResponseList response =
api.postBarcodeRecognizeFromUrlOrContent(
type,checksumValidation,detectEncoding,preset,rectX,rectY,rectWidth,rectHeight,
stripFNC,timeout,medianSmoothingWindowSize,allowMedianSmoothing,allowComplexBackground,
allowDatamatrixIndustrialBarcodes,allowDecreasedImage,allowDetectScanGap,
allowIncorrectBarcodes,allowInvertImage,allowMicroWhiteSpotsRemoving,allowOneDFastBarcodesDetector,
allowOneDWipedBarsRestoration,allowQRMicroQrRestoration,allowRegularImage,allowSaltAndPepperFiltering,
allowWhiteSpotsRemoving,regionLikelihoodThresholdPercent,scanWindowSizes,similarity,skipDiagonalSearch,
australianPostEncodingTable,rectangleRegion,url,image);
assertNotNull(response);
assertFalse(response.getBarcodes().isEmpty());
BarcodeResponse barcode = response.getBarcodes().get(0);
assertEquals(DecodeBarcodeType.CODE11.getValue(), barcode.getType());
assertEquals("1234567812", barcode.getBarcodeValue());

画像 3:- 2D バーコードのプレビュー。
上記のコードを上記の画像で実行すると、レスポンス本文は出力を次のようにレンダリングします。
レスポンスボディ
{ "barcodes": [ { "barcodeValue": "12345678", "type": "Code39Standard", "region": [ { "x": **28**, "y": **3** }, { "x": **222**, "y": **3** }, { "x": **222**, "y": **74** }, { "x": **28**, "y": **74** } ], "checksum": "" } ] }
結論
この記事では、Java REST API を使用してバーコード スキャナー アプリを開発する手順を学びました。同様に、API を使用すると、画像ファイルから QR コード リーダーを実装することもできます。 Java SDK を使用する以外に、cURL コマンドを使用してオンラインでバーコードをスキャンするオプションもあります。追加のソフトウェアのダウンロードやインストールは必要ありません。 API の使用中に問題が発生した場合は、無料の製品サポート フォーラム からお気軽にお問い合わせください。
関連記事
また、次のリンクにアクセスして詳細を確認することをお勧めします。