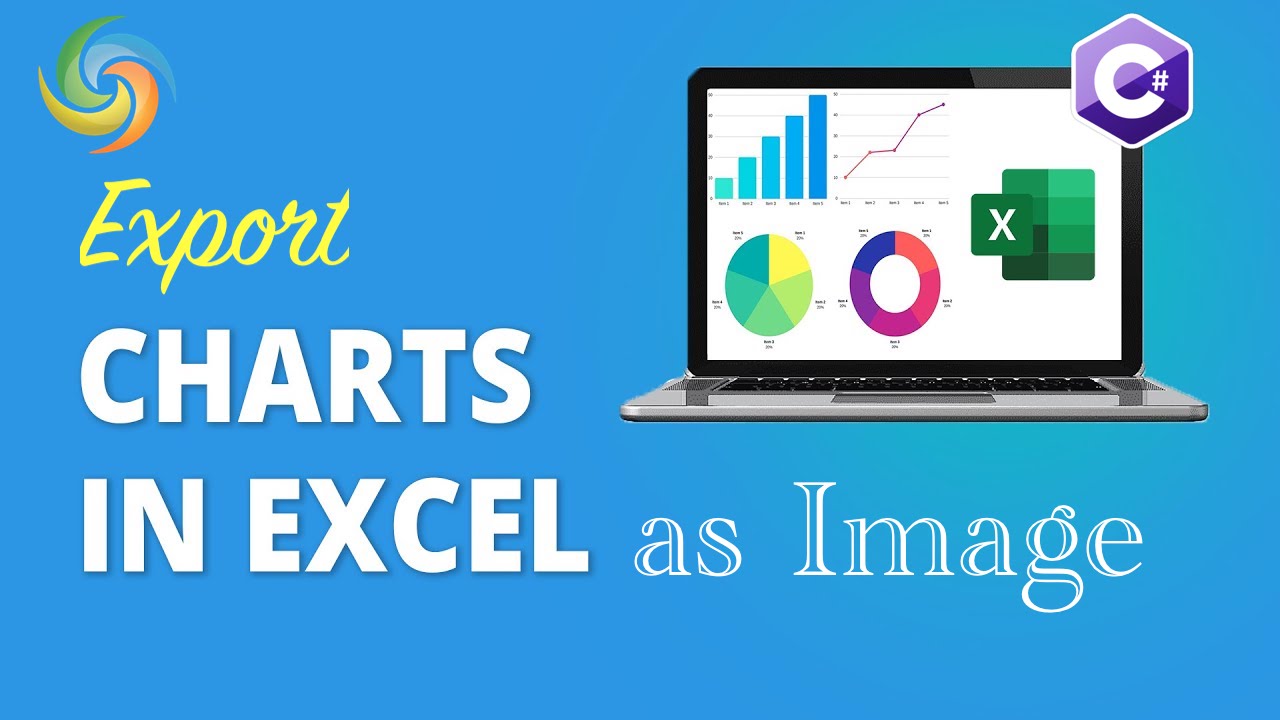
Export Excel chart as image (JPG, PNG) using C#
In the world of data analysis, visualizing data using charts and graphs is an essential part of presenting complex information in an easily digestible manner. Excel is a popular tool used by many data analysts to create charts and graphs, but sometimes it is necessary to export these charts as images to be used in reports, presentations, or other documents. Exporting charts as images also has the benefit of maintaining the formatting and visual appeal of the original chart, even when it is inserted into a document created in a different program. In this article, we will explore how to export Excel charts as images using C# programming language, providing you with the benefits of clear and concise data visualization.
- Excel Processing API
- Export Excel Chart as Image using C#
- Save Excel Chart as Image using cURL Commands
Excel Processing API
Aspose.Cells Cloud is a powerful cloud-based platform that offers a wide range of features for working with Excel files. Whether you need to manipulate data, perform calculations, or generate reports, Aspose.Cells Cloud has you covered. One particularly useful feature is the ability to export Excel charts as images. So in order to export an Excel chart as an image in .NET, we are going to use Aspose.Cells Cloud SDK for .NET. This cloud-based solution provides a RESTful API that allows you to convert Excel charts to JPG, PNG, BMP etc.
Search Aspose.Cells-Cloud in NuGet packages manager and click the “Add Package” button. Furthermore, we also need to create an account over Dashboard using a valid email address.
Export Excel Chart as Image using C#
Please use the following code snippets to export Excel chart as Image using C# .NET. In this example, we are going to save an Excel graph to JPG.
Given below are the details of above code snippet:
CellsApi cellsInstance = new CellsApi(clientID, clientSecret);
Create an object of CellsApi class while passing client credentials as arguments.
cellsInstance.UploadFile(input_Excel, File.OpenRead(input_Excel));
Read the Excel file and upload to cloud storage.
var response = cellsInstance.CellsChartsGetWorksheetChart(input_Excel, sheetName, chartNumber, imageFormat, null);
Call the API to export Excel chart as an Image. We have passed ‘JPEG’ as resultant image format.
The API supports the following image formats PNG/TIFF/JPEG/GIF/EMF/BMP.
using (var fileStream = new FileStream("resultant.jpg", System.IO.FileMode.OpenOrCreate, FileAccess.Write))
{
response.Seek(0, SeekOrigin.Begin);
response.CopyTo(fileStream);
}
Save the JPG image to local drive.
The input Excel used in the above example can be downloaded from source.xlsx.
Save Excel Chart as Image using cURL Commands
Exporting an Excel chart as an image can also be done using the Aspose.Cells Cloud and cURL command. With this option, you can quickly integrate chart-to-image conversion functionality into your application without the need for complex coding. By simply sending a request to the Aspose.Cells Cloud API using a cURL command, you can convert an Excel chart to a variety of image formats.
First, we need to install cURL on our system and then generate an accessToken based on your client credentials:
curl -v "https://api.aspose.cloud/connect/token" \
-X POST \
-d "grant_type=client_credentials&client_id=bb959721-5780-4be6-be35-ff5c3a6aa4a2&client_secret=4d84d5f6584160cbd91dba1fe145db14" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "Accept: application/json"
Secondly, use the following command to upload the input Excel to cloud storage:
curl -v "https://api.aspose.cloud/v3.0/cells/storage/file/{filePath}" \
-X PUT \
-F file=@{localFilePath} \
-H "Content-Type: multipart/form-data" \
-H "Authorization: Bearer {accessToken}"
Replace
{filePath}
with the path where you want to store the file in the cloud storage,{localFilePath}
with the path of an Excel on your local system, and{accessToken}
with your Aspose Cloud access token (generated above).
Now, we need to execute the following command to compress save Excel graph as image:
curl -v "https://api.aspose.cloud/v3.0/cells/{excelFile}/worksheets/Sheet1/charts/0?format={format}" \
-X GET \
-H "accept: multipart/form-data" \
-H "authorization: Bearer {accessToken}" \
-o "Resultant.jpg"
Replace
{excelFile}
with the name of Excel workbook available in cloud storage. Replace{format}
with desired image format, i.e. PNG/TIFF/JPEG/GIF/EMF/BMP. Now replace{accessToken}
with the access token generated above . The -o parameter is used to download the output on local drive.
Concluding Remarks
In conclusion, exporting Excel charts as images can be a very useful feature when you need to share or publish your data in a visual format. Aspose.Cells Cloud provides a comprehensive solution for this task, offering a wide range of tools and APIs that can be used to easily export Excel charts as images. The platform’s integration with cURL command makes it possible to automate this process, making it even more efficient and time-saving. Whether you are working on a small project or a large-scale data analysis, Aspose.Cells Cloud can help you achieve your goals quickly and easily.
Useful Links
Recommended Articles
Please visit the following links to learn more about: