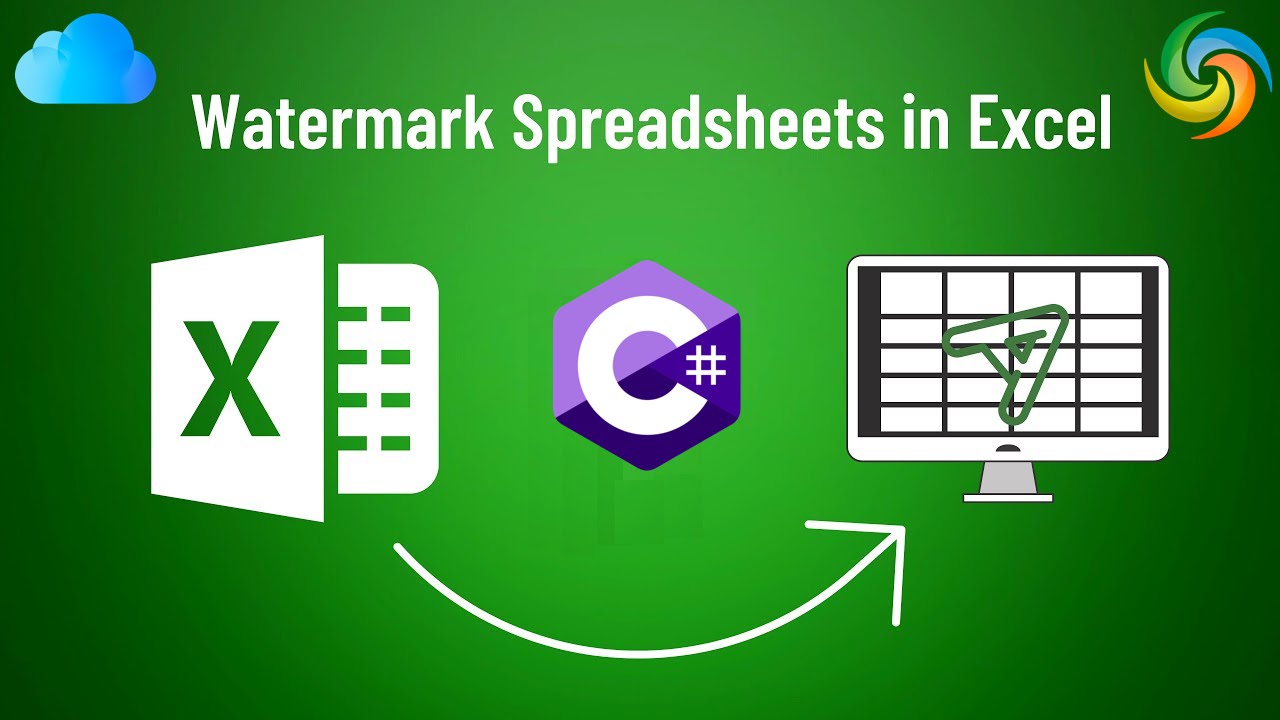
如何使用 C# 在 Excel(XLS, XLSX) 中插入水印
Excel 是一種功能強大的工具,廣泛用於管理和分析數據。由於它主要以其數字和數據處理功能而聞名,它還提供了許多有用的格式化和演示工具。其中一種工具是能夠插入水印,可用於將背景圖像或文本添加到 Excel 工作表。水印可用於向文檔添加品牌元素,指示文檔的狀態或版本,或添加一層保護以防止未經授權的複製或分發。在本文中,我們將探討如何使用 C# 在 Excel 中添加和刪除水印,為那些想要增強 Excel 文檔的視覺吸引力並保護其寶貴內容的用戶提供分步指南。
Excel 水印 API
Aspose.Cells Cloud 提供了一種在雲中處理 Excel 文檔的簡單高效的方式,使您能夠簡化工作流程並自動執行許多與 Excel 相關的任務。由於其跨平台兼容性、無縫集成、強大的安全性和成本效益,它是在雲中處理 Excel 文件的絕佳選擇。此外,這個強大的 API 允許您以編程方式對 Excel 文檔執行各種操作,包括添加和刪除水印。
現在,為了使用 C# .NET 在 Excel 中插入水印,我們需要在我們的項目中添加 Aspose.Cells Cloud SDK for .NET 的引用。因此,在 NuGet 包管理器中搜索 Aspose.Cells-Cloud 並單擊“添加包”按鈕。此外,我們還需要使用有效的電子郵件地址在 Dashboard 上創建一個帳戶。
使用 C# 向 Excel 添加水印
讓我們快速瀏覽一下用於向 Excel 工作簿添加水印的 C# .NET 代碼片段。
// 如需完整示例和數據文件,請訪問
https://github.com/aspose-cells-cloud/aspose-cells-cloud-dotnet/
// 從 https://dashboard.aspose.cloud/ 獲取客戶端憑證
string clientSecret = "4d84d5f6584160cbd91dba1fe145db14";
string clientID = "bb959721-5780-4be6-be35-ff5c3a6aa4a2";
// 在傳遞 ClientID 和 ClientSecret 時創建 CellsApi 實例
CellsApi cellsInstance = new CellsApi(clientID, clientSecret);
// 從本地驅動器輸入 Excel 工作簿
string input_Excel = "input.xls";
// 用作水印的圖像
string imageFile = "Landscape.jpg";
// 讀取輸入圖像到流實例
var imageStream = System.IO.File.OpenRead(imageFile);
try
{
// 讀取 Excel 工作簿並上傳到雲存儲
cellsInstance.UploadFile(input_Excel, File.OpenRead(input_Excel));
// 創建內存流實例
var memoryStream = new MemoryStream();
// 使用 .CopyTo() 方法並將當前文件流寫入內存流
imageStream.CopyTo(memoryStream);
// 將流轉換為數組
byte[] imageBytes = memoryStream.ToArray();
// 為 Excel 工作簿添加水印
var response = cellsInstance.CellsWorkbookPutWorkbookBackground(input_Excel, imageBytes, null);
// 如果連接成功則打印成功消息
if (response != null && response.Equals("OK"))
{
Console.WriteLine("Excel Watermark operation successful !");
Console.ReadKey();
}
}
catch (Exception ex)
{
Console.WriteLine("error:" + ex.Message + "\n" + ex.StackTrace);
}
以下是上述代碼片段的詳細信息:
CellsApi cellsInstance = new CellsApi(clientID, clientSecret);
創建一個 CellsApi 對象,同時將客戶端憑據作為參數傳遞。
var imageStream = System.IO.File.OpenRead(imageFile);
將輸入圖像讀取到 FileStream 實例。
cellsInstance.UploadFile(input_Excel, File.OpenRead(input_Excel));
將輸入的 Excel 上傳到雲存儲。
var memoryStream = new MemoryStream();
imageStream.CopyTo(memoryStream);
byte[] imageBytes = memoryStream.ToArray();
輸入 FileStream 被轉換為 ByteArray。
var response = cellsInstance.CellsWorkbookPutWorkbookBackground(input_Excel, imageBytes, null);
最後,我們調用API為Excel添加水印,並將生成的Workbook保存到雲存儲。
上例中使用的輸入Excel和Image文件可以從input.xls和[Landscape.jpg](https://media.photographycourse.net/wp-content/uploads/ 2014/11/08164934/Landscape-Photography-steps.jpg)分別。
使用 C# 去除 Excel 水印
借助 Aspose.Cells Cloud,從 Excel 文檔中去除水印既快速又直接,使您能夠簡化工作流程並自動執行許多與 Excel 相關的任務。當您需要更新或替換現有水印,或者您想要完全刪除它時,此任務很有用。使用 Aspose.Cells Cloud API,您可以輕鬆地從所有 Excel 工作表中刪除水印。然後,API 將從指定的工作表中刪除水印,文檔的其餘部分保持不變。
// 如需完整示例和數據文件,請訪問
https://github.com/aspose-cells-cloud/aspose-cells-cloud-dotnet/
// 從 https://dashboard.aspose.cloud/ 獲取客戶端憑證
string clientSecret = "4d84d5f6584160cbd91dba1fe145db14";
string clientID = "bb959721-5780-4be6-be35-ff5c3a6aa4a2";
// 在傳遞 ClientID 和 ClientSecret 時創建 CellsApi 實例
CellsApi cellsInstance = new CellsApi(clientID, clientSecret);
// 在本地驅動器上輸入帶有水印的 Excel 工作簿
string input_Excel = "input.xls";
try
{
// 讀取 Excel 工作簿並上傳到雲存儲
cellsInstance.UploadFile(input_Excel, File.OpenRead(input_Excel));
// 調用 API 從所有 Excel 工作表中刪除水印
var response = cellsInstance.CellsWorkbookDeleteWorkbookBackground(input_Excel, null);
// 如果連接成功則打印成功消息
if (response != null && response.Equals("OK"))
{
Console.WriteLine("Watermarks removed successfully from Excel !");
Console.ReadKey();
}
}
catch (Exception ex)
{
Console.WriteLine("error:" + ex.Message + "\n" + ex.StackTrace);
}
在上面的代碼片段中,以下代碼行負責從 Excel 工作簿中刪除水印圖像。
var response = cellsInstance.CellsWorkbookDeleteWorkbookBackground(input_Excel, null);
使用 cURL 命令設置 Excel 背景圖像
Aspose.Cells Cloud 提供簡單易用的 REST API,允許您將 Excel 文檔的水印功能無縫集成到您的工作流程中。此外,在 cURL 命令的幫助下,我們可以自動執行此操作並簡化我們與 Excel 相關的任務。現在,為了添加水印,我們需要向 Aspose.Cells Cloud API 發送一個 cURL POST 請求,其中水印設置和 Excel 文檔文件作為參數。
但是,首先我們需要在系統上安裝 cURL,然後根據客戶端憑據生成 accessToken:
curl -v "https://api.aspose.cloud/connect/token" \
-X POST \
-d "grant_type=client_credentials&client_id=bb959721-5780-4be6-be35-ff5c3a6aa4a2&client_secret=4d84d5f6584160cbd91dba1fe145db14" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "Accept: application/json"
其次,使用以下命令將輸入的Excel上傳到雲存儲:
curl -v "https://api.aspose.cloud/v3.0/cells/storage/file/{filePath}" \
-X PUT \
-F file=@{localFilePath} \
-H "Content-Type: multipart/form-data" \
-H "Authorization: Bearer {accessToken}"
將
{filePath}
替換為您要在雲存儲中存儲文件的路徑,將{localFilePath}
替換為本地系統上 Excel 的路徑,並將{accessToken}
替換為您的 Aspose Cloud 訪問令牌(上面生成)。
最後,執行以下命令在在線Excel工作簿中插入水印:
curl -v "https://api.aspose.cloud/v3.0/cells/{excelFile}/background" \
-X PUT \
-H "accept: multipart/form-data" \
-H "authorization: Bearer {accessToken}" \
-H "Content-Type: multipart/form-data" \
-d "File":{"watermarkImage"}
將
{excelFile}
替換為雲存儲中輸入的 Excel 文件的名稱 用上面生成的訪問令牌替換{accessToken}
將{watermarkImage}
替換為本地驅動器上可用的光柵圖像
- 操作成功後,帶水印的Excel將保存在同一個雲存儲中。
結束語
總體而言,在 Excel 文檔中添加和刪除水印有助於保護您的數據並保持文檔完整性。 Aspose.Cells Cloud 提供了一個強大的解決方案,以簡單和流線型的方式完成這些任務。通過使用 Aspose.Cells Cloud API 和 cURL 命令,您可以輕鬆地自動化這些過程並將它們集成到您現有的工作流程中。 Aspose.Cells Cloud 具有文檔轉換、格式化和操作等附加功能,是在雲中管理 Excel 文檔的寶貴工具。
有用的鏈接
推薦文章
請訪問以下鏈接以了解更多信息: