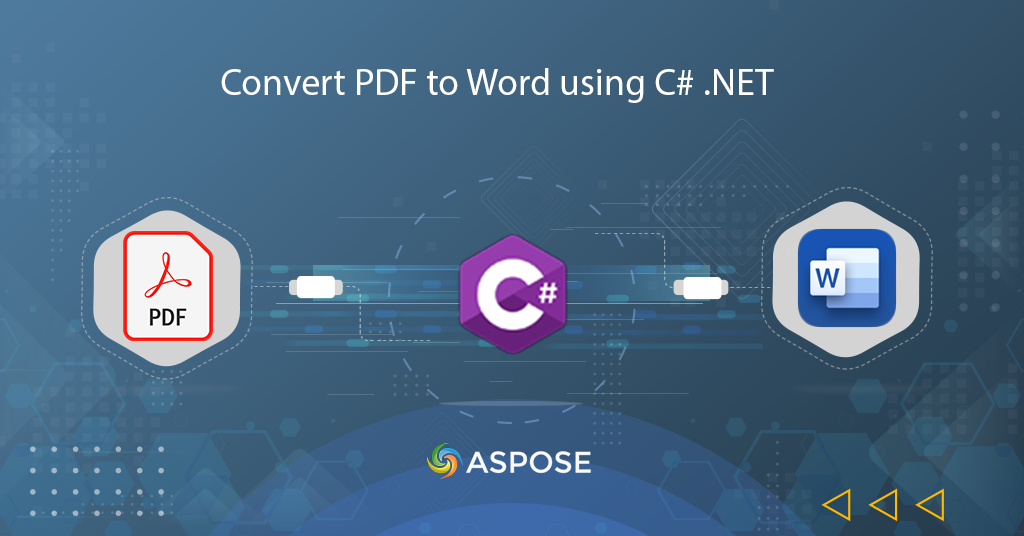
Convert PDF to Word using C# .NET
A large number of documents are produced in Portable Document Format (PDF) because it preserves document formatting on all platforms (desktop or mobile). However, we cannot directly modify the PDF files and for that purpose, we need to use some PDF manipulation software which includes installation and licensing costs. Therefore, one of the viable solutions is the conversion of PDF to Word format.
- PDF to Word Conversion API
- PDF to DOC in C#
- Convert to Word - Save Output in Cloud Storage
- PDF to Word DOC using cURL Commands
PDF to Word Conversion API
Aspose.PDF Cloud is an amazing REST-based API offering the capabilities to create, manipulate and render PDF
files into DOC, DOCX, XLSX, PPTX, HTML, etc. Owing to its REST architecture, it can be used on any platform including desktop, web, mobile, and any operating system such as Windows, macOS, Linux, etc. So in order to convert PDF to Word within .NET application, Aspose.PDF Cloud SDK for .NET can be used. Now please execute the following command in the NuGet package manager to install the latest release of SDK.
Install-Package Aspose.Pdf-Cloud
Referencing within VisualStudio
We can also add the SDK directly inside the Visual Studio project. Therefore, expand the project tree in Solution Explorer, right-click the Packages folder, and select Manage NuGet Packages… option from the context menu. Search Aspose.PDF Cloud in the search field, enable the checkbox beside the package name and click Add Package button
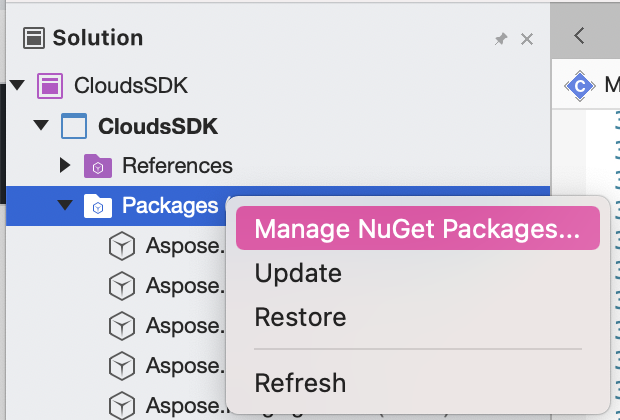
Image 1:- Manage NuGet packages.
In case you do not have an account over Aspose.Cloud dashboard, please create a free account using your existing GitHub or Google account or, click on the Create a new Account button. Obtain your personalized Client Credentials as they will be required in following sections.
PDF to DOC in C#
Please follow the steps specified below to perform the conversion of PDF files to Word document (DOC) format in the C# .NET application. After the conversion, the output is stored in a steam instance which can be later saved on the local system.
- Firstly, define Client ID and Client Secret details using string objects
- Secondly, initialize the PdfApi object while passing Client ID and Client Secret details as arguments
- Thirdly, read the file content and upload it to Cloud storage using the UploadFile(..) method of PdfApi
- Now we need to call the GetPdfInStorageToDoc(…) method which takes PDF file reference from cloud storage and other optional parameters such as format, maxDistanceBetweenTextLines, conversion mode, etc.
- Since the output is in steam, so we can use File.Create to save it over the system drive

Image 1:- PDF to DOC conversion preview.
Please visit the following links to download HtmlExample1.pdf and Converted.docx.
Convert PDF to Word - Save Output in Cloud Storage
In this section, we are going to discuss the process of a loading PDF documents from Cloud storage, performing their conversion to Word format and saving the output in Cloud storage. Please follow the instructions specified below to accomplish this task:
- First, create an instance of PdfApi by passing Client ID and Client secret details as arguments
- Load the PDF file from the local system and upload it to cloud storage using the UploadFile(..) method of PdfApi (this is an optional step and can be used if you do not have any PDF already in Cloud storage)
- Create. a string variable defining the resultant file name
- Finally, call the PutPdfInStorageToDoc(..) which takes input PDF name, output file name and other optional parameters
PDF to Word DOC using cURL Commands
The cURL commands are an amazing mechanism for accessing the REST APIs. So in the following section, we are going to perform the PDF to Word Doc conversion using the cURL command. However, as a pre-requisite, the first step is to generate a JSON Web Token (JWT) based on ClientID and ClientSecret details retrieved from Aspose.Cloud dashboard. Please execute the following command in the terminal to generate the JWT token.
curl -v "https://api.aspose.cloud/connect/token" \
-X POST \
-d "grant_type=client_credentials&client_id=4ccf1790-accc-41e9-8d18-a78dbb2ed1aa&client_secret=caac6e3d4a4724b2feb53f4e460eade3" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "Accept: application/json"
Once we have the JWT token, we can execute the following command to perform conversion of PDF file already available in cloud storage and also save the output file in cloud storage.
curl -X PUT "https://api.aspose.cloud/v3.0/pdf/HtmlExample1.pdf/convert/doc?outPath=converted.docx&addReturnToLineEnd=true&format=DocX&mode=Textbox" \
-H "accept: application/json" \
-H "authorization: Bearer <JWT Token>"
Conclusion
This article has explained the steps for developing a PDF to Word Converter using Aspose.PDF Cloud. We have explored the options to convert PDF to DOC using C# code snippet as well as to convert PDF to Word using cURL commands. Apart from conversion, it also provides other exciting features and their details can be found over Aspose.PDF Cloud Features.
Since our Cloud SDKS are developed under MIT License, so their complete code snippet can be downloaded from GitHub. In case you encounter any issue while using the API or you have any related query, please feel free to contact via Free Support forum.
Related articles
We also recommend visiting following links to learn more about