With the release of Aspose.Imaging Cloud 20.5, we are pleased to announce the feature of object detection in images. It enables the users to detect object boundaries on an input image based on their labels and probabilities. The current implementation supports a single shot detector method to recognize objects where the model is trained using COCO 2017 dataset. The SSD approach discretizes the output space of bounding boxes into a set of default boxes over different aspect ratios and scales per feature map location.
Aspose.Imaging Cloud performs object detection based on the following 4 methods:
- Detect objects on an existing image and return results as a JSON object
- Detect objects on an existing image and return results as an image
- Upload an image, detect objects on it, and return results as a JSON object
- Upload an image, detect objects on it, and return results as an image
In this section, we are going to discuss the following sections in further details
Detect object Bounds
This approach detects objects on an existing image and returns the result as a JSON object.
Request query parameters:
- name (string, required): image name. Currently, we support 3 image formats: BMP, JPEG, and JPEG 2000
- method (string, optional, default “ssd”): object detection method
- threshold (number, optional, [0 - 100], default 50): minimum detected objects probability in percentage that will be included in the result
- includeLabel (boolean, optional, default false): whether to include detected object labels in the response
- includeScore (boolean, optional, default false): whether to include detected object probabilities in the response
- folder (string, optional): folder
- storage (string, optional): storage
Object Detection using cURL Command
Aspose.Imaging Cloud can also be accessed using the cURL commands. The following command shows how a cURL command can be used to detect an object and get a response as a JSON object.
The following sample image is being used to detect the object.
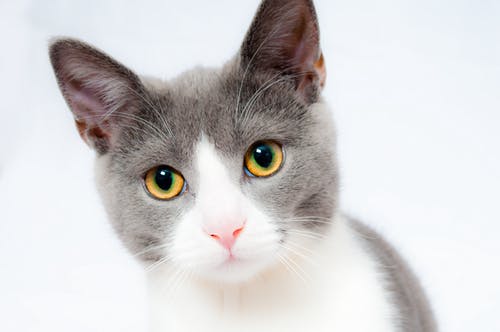
Image 1:- Source image
curl "https://api.aspose.cloud/v3.0/imaging/ai/objectdetection/cat-pet-animal-domestic-104827.jpeg/bounds?method=ssd&threshold=50&includeLabel=true&includeScore=true" \
-X GET \
-H "accept: application/json" \
-H "authorization: Bearer <jwt token>"
Request URL
https://api.aspose.cloud/v3.0/imaging/ai/objectdetection/cat-pet-animal-domestic-104827.jpeg/bounds?method=ssd&threshold=50&includeLabel=true&includeScore=true
Response Body
{
"detectedObjects": [
{
"label": "cat",
"score": 0.9450986,
"bounds": {
"x": 43,
"y": 4,
"width": 401,
"height": 323
}
}
]
}
Response Schema
{
"type": "object",
"properties": {
"detectedObjects": {
"type": "array",
"items": [
{
"type": "object",
"properties": {
"score": {
"type": "number"
},
"label": {
"type": "string"
},
"bounds": {
"type": "object",
"properties": {
"x": {
"type": "number"
},
"y": {
"type": "number"
},
"width": {
"type": "number"
},
"height": {
"type": "number"
}
},
"required": [
"x",
"y",
"width",
"height"
]
}
},
"required": [
"score",
"label",
"bounds"
]
}
]
}
},
"required": [
"detectedObjects"
]
}
Object Detection in Images using C#
Please try using the following code snippet for object detection in images using the C# code snippet.
C# .NET code snippet
Detect Object Bounds and Return as Image
Upload an image, detect objects, draw bounds around them and, return results as an image.
Request query parameters:
- name (string, required): image name. Currently, 3 image formats are supported: bmp, jpg, jpeg, and jpeg2000
- method (string, optional, [“ssd”], default “ssd”): object detection method
- threshold (number, optional, [0 - 100], default 50): minimum objects’ probability in percents that will be included in the result
- includeLabel (boolean, optional, default false): whether to include detected object labels in the response
- includeScore (boolean, optional, default false): whether to include detected object probabilities in the response
- color (string, optional): the custom color of the detected object bounds and info. If equals to null, objects of different labels have bounds of different colors
- folder (string, optional): folder
- storage (string, optional): storage
Using cURL command to determine objects
Aspose.Imaging Cloud can also be accessed through cURL commands. However, as a pre-requisite, you need to first generate a JWT access token based on your client credentials. Please execute the following command to generate the JWT token.
curl -v "https://api.aspose.cloud/connect/token" \
-X POST \
-d "grant_type=client_credentials&client_id=4ccf1790-accc-41e9-8d18-a78dbb2ed1aa&client_secret=caac6e3d4a4724b2feb53f4e460eade3" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "Accept: application/json"
Now execute the following command to detect object bounds and return them as an image.
curl -v -X GET "https://api.aspose.cloud/v3.0/imaging/ai/objectdetection/dog-and-cat-cover.jpg/visualbounds?method=ssd&threshold=50&includeLabel=false&includeScore=false&color=Red" \
-H "accept: application/json" \
-H "authorization: Bearer <JWT Token>"
Request URL
https://api.aspose.cloud/v3.0/imaging/ai/objectdetection/dog-and-cat-cover.jpg/visualbounds?method=ssd&threshold=50&includeLabel=false&includeScore=false&color=Red
In the above request, notice Red is specified as a highlight color.
C#.NET code snippet
The following code snippet shows steps to load an image file containing two objects (Dog and Cat). Both of these objects are identified using Aspose.Imaging Cloud API. The resultant images with highlighted objects are saved on the system drive.
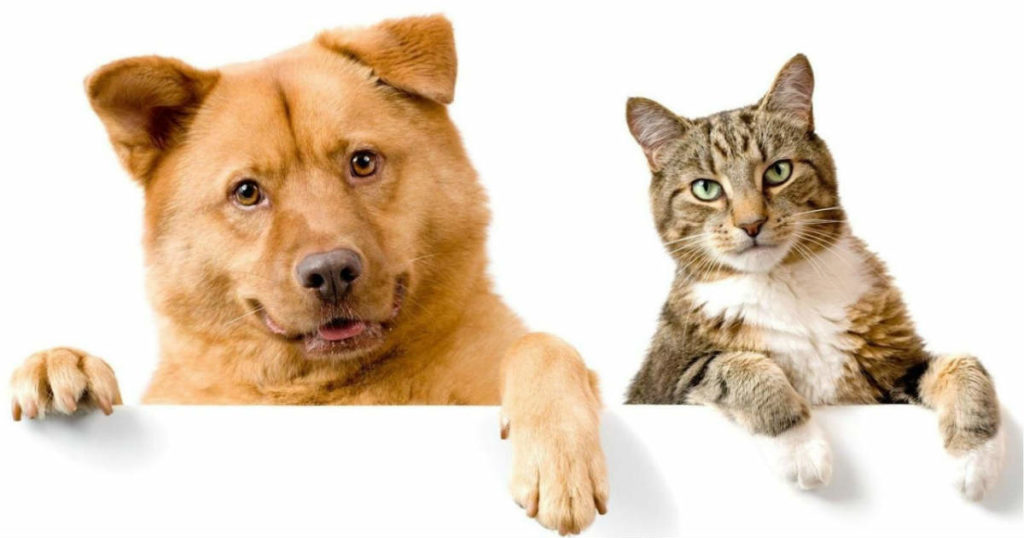
Image 2:- Input image with Cat and Dog
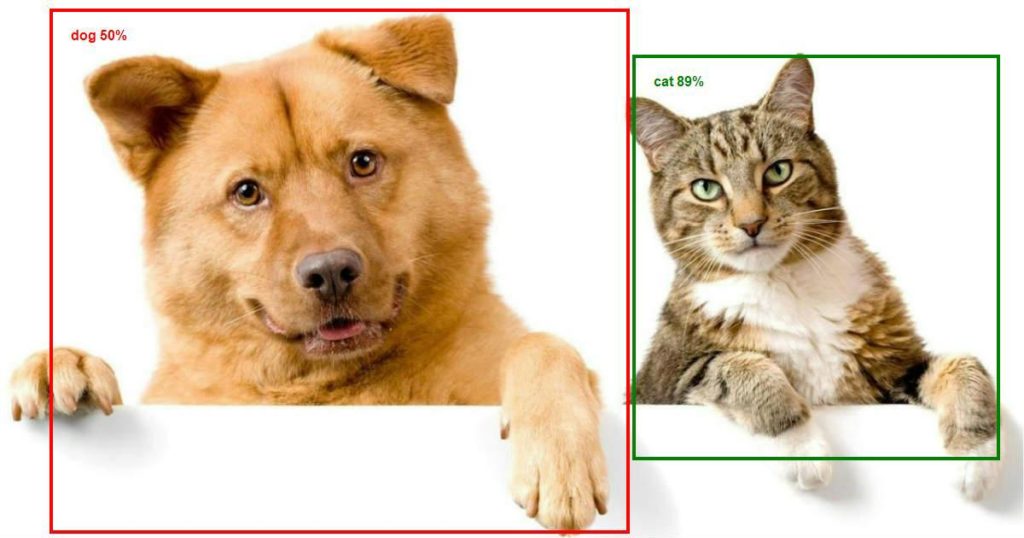
Image 3:- Processed image highlighting identified objects
For more information on Image search, please visit Reverse Image Search.
Conclusion
In this article, we have learned the capabilities of Aspose.Imaging Cloud for Object Detection in Images. In order to identify objects in images, you may either use the code snippet or use the cURL commands. Furthermore, in order to facilitate our customers, we have created programming language-specific SDKs and in the above article, we have explored the features of Aspose.Imaging Cloud SDK for .NET for object detection. The complete source code of SDK is available for download over GitHub.
Related Articles
We recommend visiting the following links to learn more about: